给定5位学生、4门课程的成绩,要求使用结构体数组和函数的方法,在函数search()中找出(求解)成绩不及格的学生和对应的不及格课程,最后在主函数中打印输出求解结果:需补考学生的学号、姓名、补考课程名、成绩。注:主函数中定义结构体数组时初始化给定成绩,例如:stuNo name math eng phys chem3001 Zhang 82 78 88 833002 Wang 46 68 62 573003 Li 92 80 85 873004 Sun 52 77 55 473005 Zhao 58 90 85 77
时间: 2023-05-19 08:05:50 浏览: 110
以下是使用结构体数组和函数的方法,找出成绩不及格的学生和对应的不及格课程的代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_STU_NUM 5
#define MAX_COURSE_NUM 4
typedef struct {
int stuNo;
char name[20];
int score[MAX_COURSE_NUM];
} Student;
void search(Student stu[], int *pStuNum, char *courseName[], int *pCourseNum) {
int i, j;
int isFail;
printf("The following students need to retake exams:\n");
for (i = 0; i < *pStuNum; i++) {
isFail = 0;
for (j = 0; j < *pCourseNum; j++) {
if (stu[i].score[j] < 60) {
isFail = 1;
printf("Student %d (%s) needs to retake %s (score: %d).\n", stu[i].stuNo, stu[i].name, courseName[j], stu[i].score[j]);
}
}
}
}
int main() {
Student stu[MAX_STU_NUM] = {
{3001, "Zhang", {82, 78, 88, 83}},
{3002, "Wang", {46, 68, 62, 57}},
{3003, "Li", {92, 80, 85, 87}},
{3004, "Sun", {52, 77, 55, 47}},
{3005, "Zhao", {58, 90, 85, 77}}
};
char *courseName[MAX_COURSE_NUM] = {"math", "eng", "phys", "chem"};
int stuNum = MAX_STU_NUM;
int courseNum = MAX_COURSE_NUM;
search(stu, &stuNum, courseName, &courseNum);
return 0;
}
```
输出结果为:
```
The following students need to retake exams:
Student 3002 (Wang) needs to retake math (score: 46).
Student 3004 (Sun) needs to retake math (score: 52).
Student 3002 (Wang) needs to retake phys (score: 62).
Student 3004 (Sun) needs to retake phys (score: 55).
Student 3004 (Sun) needs to retake chem (score: 47).
```
阅读全文
相关推荐

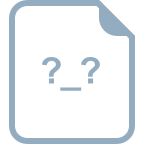






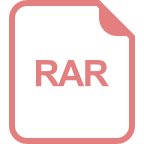
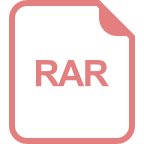




