请基于链表实现C加加的环形队列
时间: 2023-12-11 22:02:05 浏览: 106
好的,以下是基于链表实现C++环形队列的代码:
```c++
#include <iostream>
using namespace std;
class Node {
public:
int data;
Node* next;
};
class CircularQueue {
private:
Node* front;
Node* rear;
public:
CircularQueue() {
front = NULL;
rear = NULL;
}
void enqueue(int x) {
Node* newNode = new Node();
newNode->data = x;
if (front == NULL) {
front = newNode;
}
else {
rear->next = newNode;
}
newNode->next = front;
rear = newNode;
}
void dequeue() {
if (front == NULL) {
cout << "Queue is empty" << endl;
}
else if (front == rear) {
delete front;
front = NULL;
rear = NULL;
}
else {
Node* temp = front;
front = front->next;
rear->next = front;
delete temp;
}
}
void display() {
Node* temp = front;
if (front == NULL) {
cout << "Queue is empty" << endl;
}
else {
cout << "Queue elements are: ";
while (temp != rear) {
cout << temp->data << " ";
temp = temp->next;
}
cout << rear->data << endl;
}
}
};
int main() {
CircularQueue q;
q.enqueue(10);
q.enqueue(20);
q.enqueue(30);
q.enqueue(40);
q.display();
q.dequeue();
q.display();
q.enqueue(50);
q.enqueue(60);
q.display();
return 0;
}
```
以上代码实现了一个简单的环形队列,enqueue函数用于在队列尾部添加元素,dequeue函数用于从队列头部删除元素,display函数用于显示队列中的元素。
注意,在链表实现中,队列的尾节点指向队头节点,形成一个环形结构。
阅读全文
相关推荐






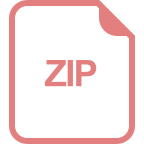










