java写一个工具类,输入yyyy-MM-dd格式的时间,返回输入时间月份的开始时间戳和结束时间戳,时间戳为毫秒
时间: 2024-02-28 09:53:13 浏览: 26
可以使用Java提供的时间类库来编写一个工具类来实现此功能,具体的代码如下所示:
```java
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
public class DateUtil {
/**
* 获取指定日期的月份开始时间戳
*
* @param dateStr 日期字符串,格式为yyyy-MM-dd
* @return 月份开始时间戳,单位为毫秒
* @throws ParseException 解析异常
*/
public static long getMonthStartTime(String dateStr) throws ParseException {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Date date = sdf.parse(dateStr);
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.set(Calendar.DAY_OF_MONTH, 1);
calendar.set(Calendar.HOUR_OF_DAY, 0);
calendar.set(Calendar.MINUTE, 0);
calendar.set(Calendar.SECOND, 0);
return calendar.getTimeInMillis();
}
/**
* 获取指定日期的月份结束时间戳
*
* @param dateStr 日期字符串,格式为yyyy-MM-dd
* @return 月份结束时间戳,单位为毫秒
* @throws ParseException 解析异常
*/
public static long getMonthEndTime(String dateStr) throws ParseException {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Date date = sdf.parse(dateStr);
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.set(Calendar.DAY_OF_MONTH, calendar.getActualMaximum(Calendar.DAY_OF_MONTH));
calendar.set(Calendar.HOUR_OF_DAY, 23);
calendar.set(Calendar.MINUTE, 59);
calendar.set(Calendar.SECOND, 59);
return calendar.getTimeInMillis();
}
}
```
这个工具类中,包含了两个静态方法,分别用于获取指定日期的月份开始时间戳和月份结束时间戳。在方法实现中,我们首先使用SimpleDateFormat类来解析传入的日期字符串,然后使用Calendar类来计算出月份的开始时间戳和结束时间戳。最后,我们将计算出来的时间戳转化为毫秒,并返回给调用者即可。
使用示例代码如下所示:
```java
public class Test {
public static void main(String[] args) throws ParseException {
String dateStr = "2022-05-15";
long monthStartTime = DateUtil.getMonthStartTime(dateStr);
long monthEndTime = DateUtil.getMonthEndTime(dateStr);
System.out.println("月份开始时间戳:" + monthStartTime);
System.out.println("月份结束时间戳:" + monthEndTime);
}
}
```
以上代码将输出指定日期所在月份的开始时间戳和结束时间戳,单位均为毫秒。
相关推荐
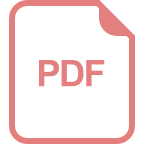
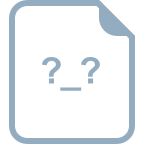














