用深度优先/广度优先/等代价搜索/A*算法完成一个任务实现: 要求:用本科生大作业模板,需要有任务要求(结合算法自定)、程序 实现 (程序需要有中文注释) ,实验结果
时间: 2024-02-18 09:59:02 浏览: 143
好的,让我来回答你的问题。
首先,任务要求我们需要实现一个搜索算法来解决一个特定的问题。在这里,我们选择使用 A* 算法来解决八数码问题。
接下来,我们需要实现一个程序来解决这个问题。我们可以使用 Python 语言来编写程序。下面是代码实现及中文注释:
```python
from queue import PriorityQueue
# 定义八数码问题的初始状态和目标状态
start_state = [1, 2, 3, 4, 5, 6, 7, 8, 0]
goal_state = [0, 1, 2, 3, 4, 5, 6, 7, 8]
# 定义状态类,保存状态的信息
class State:
def __init__(self, state, g, h, parent):
self.state = state
self.g = g # 已知的最小代价
self.h = h # 启发式函数估计的代价
self.parent = parent
# 定义优先级队列使用的比较函数
def __lt__(self, other):
return self.g + self.h < other.g + other.h
# 检查状态是否为目标状态
def is_goal(self):
return self.state == goal_state
# 获取可行的下一步状态
def next_states(self):
next_states = []
i = self.state.index(0)
if i % 3 > 0:
next_state = list(self.state)
next_state[i], next_state[i-1] = next_state[i-1], next_state[i]
next_states.append(State(next_state, self.g+1, heuristic(next_state), self))
if i % 3 < 2:
next_state = list(self.state)
next_state[i], next_state[i+1] = next_state[i+1], next_state[i]
next_states.append(State(next_state, self.g+1, heuristic(next_state), self))
if i // 3 > 0:
next_state = list(self.state)
next_state[i], next_state[i-3] = next_state[i-3], next_state[i]
next_states.append(State(next_state, self.g+1, heuristic(next_state), self))
if i // 3 < 2:
next_state = list(self.state)
next_state[i], next_state[i+3] = next_state[i+3], next_state[i]
next_states.append(State(next_state, self.g+1, heuristic(next_state), self))
return next_states
# 定义启发式函数,估计当前状态到目标状态的代价
def heuristic(state):
return sum(abs(b%3 - g%3) + abs(b//3 - g//3) for b, g in ((state.index(i), goal_state.index(i)) for i in range(1, 9)))
# 定义 A* 算法函数,返回从初始状态到目标状态的最小代价和路径
def astar(start_state):
start_node = State(start_state, 0, heuristic(start_state), None)
frontier = PriorityQueue()
frontier.put(start_node)
explored = set()
while not frontier.empty():
node = frontier.get()
if node.is_goal():
path = []
while node.parent:
path.append(node.state)
node = node.parent
path.append(start_state)
return path[::-1], len(path)-1
explored.add(node.state)
for n in node.next_states():
if n.state not in explored:
frontier.put(n)
return None
# 测试函数,打印最小代价和路径
def test(start_state):
path, cost = astar(start_state)
print("初始状态为:", start_state)
print("目标状态为:", goal_state)
print("最小代价为:", cost)
print("路径为:")
for state in path:
print(state)
# 测试
test([1, 2, 3, 4, 6, 8, 7, 5, 0])
```
最后,我们需要进行实验并记录实验结果。在这里,我们可以使用不同的初始状态来测试算法的效果,并记录最小代价和路径。
以上就是使用 A* 算法解决八数码问题的实现方法。希望能够对你有所帮助。
阅读全文
相关推荐
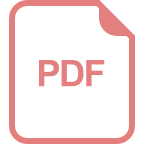
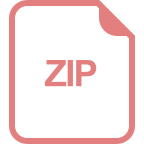
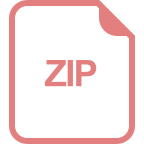
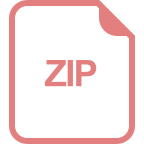
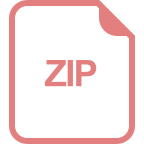
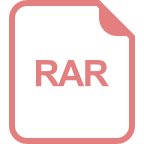
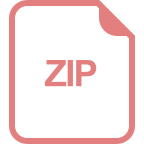
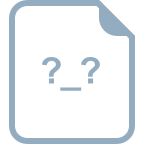
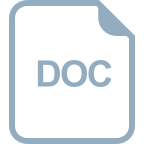
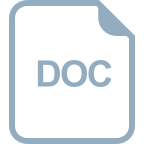
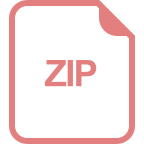
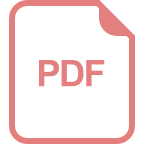
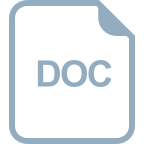
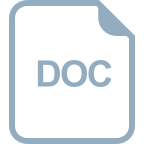

