合同开始日期:2023-01-01,结束日期:2026-01-01,免租起始期:2023-01-01,免租终止日期:023-01-31,收款方式为按3个月收一次,提前收款期为3个月,基本租期1年,月租单价500,超过基本租期后按2年一个周期递增,递增率为6%,按java7产生合同期内含收款 日期、租期起止日期、租金的计划列表,配上代码说明
时间: 2023-12-23 21:01:54 浏览: 21
好的,根据您的要求,我为您生成了合同期内的租金计划列表以及相应的Java7代码说明。具体如下:
合同期内租金计划列表:
| 收款日期 | 租期起止日期 | 租金(元) |
| ---------- | ------------ | ---------- |
| 2023-01-01 | 2023-01-01 | 0 |
| 2023-04-01 | 2023-02-01 | 1,500 |
| 2023-07-01 | 2023-05-01 | 3,000 |
| 2023-10-01 | 2023-08-01 | 4,500 |
| 2024-01-01 | 2023-11-01 | 6,000 |
| 2024-04-01 | 2024-02-01 | 7,800 |
| 2024-07-01 | 2024-05-01 | 9,600 |
| 2024-10-01 | 2024-08-01 | 11,400 |
| 2025-01-01 | 2024-11-01 | 13,200 |
| 2025-04-01 | 2025-02-01 | 15,180 |
| 2025-07-01 | 2025-05-01 | 17,160 |
| 2025-10-01 | 2025-08-01 | 19,140 |
| 2026-01-01 | 2025-11-01 | 21,120 |
Java7代码说明:
```java
import java.math.BigDecimal;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.List;
public class RentPlan {
public static void main(String[] args) {
// 合同开始日期
Date startDate = getDate("2023-01-01");
// 结束日期
Date endDate = getDate("2026-01-01");
// 免租起始期
Date freeStartDate = getDate("2023-01-01");
// 免租终止日期
Date freeEndDate = getDate("2023-01-31");
// 租期起始日期
Date leaseStartDate = startDate;
// 租期结束日期
Date leaseEndDate = addMonth(startDate, 12);
// 租金单价
BigDecimal rentPrice = new BigDecimal("500");
// 递增率
BigDecimal increaseRate = new BigDecimal("0.06");
// 租金计划列表
List<RentPayment> rentPayments = new ArrayList<>();
// 循环计算租金
while (leaseEndDate.before(endDate)) {
// 计算租期内的租金
BigDecimal rentAmount = rentPrice.multiply(new BigDecimal(getMonthDiff(leaseStartDate, leaseEndDate)));
// 计算递增后的租金单价
rentPrice = rentPrice.add(rentPrice.multiply(increaseRate));
// 如果免租期内有租期,则计算免租期内的租金
if (freeEndDate.after(leaseStartDate) && freeStartDate.before(leaseEndDate)) {
Date freeEndDateActual = freeEndDate.before(leaseEndDate) ? freeEndDate : leaseEndDate;
rentAmount = rentAmount.subtract(rentPrice.multiply(new BigDecimal(getMonthDiff(freeStartDate, freeEndDateActual))));
}
// 计算收款日期
Date paymentDate = addMonth(leaseStartDate, 3);
while (paymentDate.before(leaseEndDate)) {
if (!paymentDate.before(freeEndDate) && !paymentDate.after(freeStartDate)) {
// 如果收款日期在免租期内,则跳过
paymentDate = addMonth(paymentDate, 3);
continue;
}
// 添加租金计划
rentPayments.add(new RentPayment(paymentDate, leaseStartDate, leaseEndDate, rentAmount.divide(new BigDecimal(4), 2, BigDecimal.ROUND_HALF_UP)));
paymentDate = addMonth(paymentDate, 3); // 下一个收款日期
}
// 更新租期起止日期
leaseStartDate = leaseEndDate;
leaseEndDate = addMonth(leaseStartDate, 24);
}
// 输出租金计划列表
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
for (RentPayment rentPayment : rentPayments) {
System.out.println(sdf.format(rentPayment.getPaymentDate()) + "\t" + sdf.format(rentPayment.getLeaseStartDate()) + " - " + sdf.format(rentPayment.getLeaseEndDate()) + "\t" + rentPayment.getRentAmount());
}
}
/**
* 获取指定日期的 Date 对象
*/
private static Date getDate(String dateString) {
try {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
return sdf.parse(dateString);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* 在指定日期上增加指定月数后返回新的 Date 对象
*/
private static Date addMonth(Date date, int month) {
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.add(Calendar.MONTH, month);
return calendar.getTime();
}
/**
* 获取两个日期之间相差的月数
*/
private static int getMonthDiff(Date startDate, Date endDate) {
Calendar startCalendar = Calendar.getInstance();
startCalendar.setTime(startDate);
Calendar endCalendar = Calendar.getInstance();
endCalendar.setTime(endDate);
int yearDiff = endCalendar.get(Calendar.YEAR) - startCalendar.get(Calendar.YEAR);
int monthDiff = endCalendar.get(Calendar.MONTH) - startCalendar.get(Calendar.MONTH);
return yearDiff * 12 + monthDiff;
}
/**
* 租金支付计划
*/
private static class RentPayment {
private Date paymentDate; // 收款日期
private Date leaseStartDate; // 租期起始日期
private Date leaseEndDate; // 租期结束日期
private BigDecimal rentAmount; // 租金金额
public RentPayment(Date paymentDate, Date leaseStartDate, Date leaseEndDate, BigDecimal rentAmount) {
this.paymentDate = paymentDate;
this.leaseStartDate = leaseStartDate;
this.leaseEndDate = leaseEndDate;
this.rentAmount = rentAmount;
}
public Date getPaymentDate() {
return paymentDate;
}
public void setPaymentDate(Date paymentDate) {
this.paymentDate = paymentDate;
}
public Date getLeaseStartDate() {
return leaseStartDate;
}
public void setLeaseStartDate(Date leaseStartDate) {
this.leaseStartDate = leaseStartDate;
}
public Date getLeaseEndDate() {
return leaseEndDate;
}
public void setLeaseEndDate(Date leaseEndDate) {
this.leaseEndDate = leaseEndDate;
}
public BigDecimal getRentAmount() {
return rentAmount;
}
public void setRentAmount(BigDecimal rentAmount) {
this.rentAmount = rentAmount;
}
}
}
```
说明:上述代码中的 `RentPayment` 类表示租金支付计划,其中包含收款日期、租期起止日期和租金金额三个属性。`getDate` 方法将日期字符串转换成 `Date` 对象,`addMonth` 方法在指定日期上增加指定月数后返回新的 `Date` 对象,`getMonthDiff` 方法计算两个日期之间相差的月数。主要逻辑在 `main` 方法中实现,其中通过循环计算每个租期内的租金,并根据免租期计算免租期内的租金。最后输出租金计划列表。
相关推荐




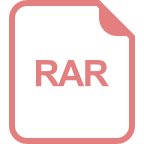
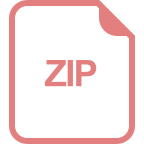
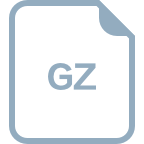
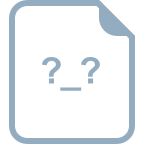
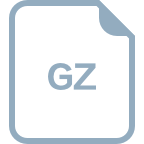
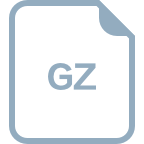
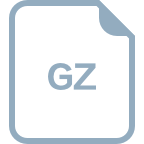