前台MainActivity如何与Mp3Service后台程序进行相互(双向)通信(源代码说明)
时间: 2023-02-07 10:21:29 浏览: 59
可以使用 Android 的跨进程通信机制,如:
- AIDL(Android Interface Definition Language)
- Messenger
- LocalBroadcastManager
其中,AIDL 是最常用的方法。
下面是使用 AIDL 进行跨进程通信的示例:
1. 在后台服务程序中定义 AIDL 接口,并在服务类中实现这个接口。
```java
// IMyAidlInterface.aidl
package com.example.myaidl;
// Declare any non-default types here with import statements
interface IMyAidlInterface {
/**
* Demonstrates some basic types that you can use as parameters
* and return values in AIDL.
*/
void basicTypes(int anInt, long aLong, boolean aBoolean, float aFloat,
double aDouble, String aString);
}
// MyService.java
public class MyService extends Service {
private final IMyAidlInterface.Stub mBinder = new IMyAidlInterface.Stub() {
@Override
public void basicTypes(int anInt, long aLong, boolean aBoolean, float aFloat,
double aDouble, String aString) {
// Do something with the values received from the client.
}
};
@Override
public IBinder onBind(Intent intent) {
return mBinder;
}
}
```
2. 在前台应用程序中创建与 AIDL 接口相对应的 Java 接口,并使用 `bindService()` 绑定服务。
```java
// IMyAidlInterface.java
package com.example.myaidl;
import android.os.IInterface;
import android.os.RemoteException;
public interface IMyAidlInterface extends IInterface {
/**
* Default implementation for IMyAidlInterface.
*/
public static class Default implements IMyAidlInterface {
@Override
public void basicTypes(int anInt, long aLong, boolean aBoolean, float aFloat,
double aDouble, String aString) throws RemoteException {
}
}
}
// MainActivity.java
public class MainActivity extends AppCompatActivity {
private IMyAidlInterface mService;
private ServiceConnection mConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
mService = IMyAidlInterface.Stub.asInterface(service);
}
@Override
public void onServiceDisconnected(ComponentName name) {
mService = null;
}
};
@Override
相关推荐
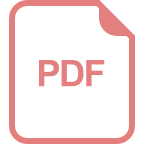
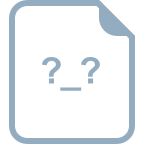
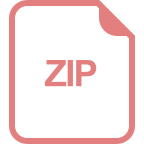














