假设我们需要实现一个基于Spring Boot和Thymeleaf的电商后台应用程序,为了更好的管理用户,需要管理所有的前台用户的注册信息。并且实现用户的封禁,解封,密码修改等功能。 【要求】 「SpringBoot」整合「Thymeleaf」实现【注册信息表】CRUD代码实现
时间: 2024-02-25 22:55:36 浏览: 66
好的,根据你的要求,以下是一个简单的基于Spring Boot和Thymeleaf的电商后台应用程序,实现了注册信息表的CRUD功能。
首先,需要创建一个名为"ecommerce"的Spring Boot项目,并添加以下依赖:
```xml
<dependencies>
<!-- Spring Boot Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Thymeleaf -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- Spring Boot Data JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- H2 Database -->
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
</dependency>
</dependencies>
```
然后,创建一个名为"User"的实体类,用于表示用户信息:
```java
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String password;
private boolean enabled;
// getters and setters
}
```
接着,创建一个名为"UserRepository"的接口,用于与数据库进行交互:
```java
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
```
然后,创建一个名为"UserController"的控制器,用于处理用户页面的请求:
```java
@Controller
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping("/users")
public String list(Model model) {
List<User> users = userRepository.findAll();
model.addAttribute("users", users);
return "users/list";
}
@GetMapping("/users/new")
public String createForm(Model model) {
model.addAttribute("user", new User());
return "users/form";
}
@PostMapping("/users/new")
public String create(@Valid User user, BindingResult result) {
if (result.hasErrors()) {
return "users/form";
}
userRepository.save(user);
return "redirect:/users";
}
@GetMapping("/users/{id}/edit")
public String updateForm(@PathVariable("id") Long id, Model model) {
User user = userRepository.findById(id).orElseThrow(() -> new IllegalArgumentException("Invalid user id: " + id));
model.addAttribute("user", user);
return "users/form";
}
@PostMapping("/users/{id}/edit")
public String update(@PathVariable("id") Long id, @Valid User user, BindingResult result) {
if (result.hasErrors()) {
user.setId(id);
return "users/form";
}
userRepository.save(user);
return "redirect:/users";
}
@GetMapping("/users/{id}/delete")
public String delete(@PathVariable("id") Long id) {
User user = userRepository.findById(id).orElseThrow(() -> new IllegalArgumentException("Invalid user id: " + id));
userRepository.delete(user);
return "redirect:/users";
}
}
```
最后,创建Thymeleaf模板,包括用户列表页面(users/list.html)和表单页面(users/form.html):
users/list.html:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>User List</title>
</head>
<body>
<h1>User List</h1>
<a href="/users/new">Create New User</a>
<table>
<thead>
<tr>
<th>ID</th>
<th>Username</th>
<th>Password</th>
<th>Enabled</th>
<th></th>
</tr>
</thead>
<tbody>
<tr th:each="user : ${users}">
<td th:text="${user.id}"></td>
<td th:text="${user.username}"></td>
<td th:text="${user.password}"></td>
<td th:text="${user.enabled}"></td>
<td>
<a th:href="@{/users/{id}/edit(id=${user.id})}">Edit</a>
<a th:href="@{/users/{id}/delete(id=${user.id})}">Delete</a>
</td>
</tr>
</tbody>
</table>
</body>
</html>
```
users/form.html:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Create/Edit User</title>
</head>
<body>
<h1>Create/Edit User</h1>
<form th:object="${user}" th:action="@{/users/{id}/edit(id=${user.id})}" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" th:field="*{username}" required>
<span th:if="${#fields.hasErrors('username')}" th:errors="*{username}"></span>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" th:field="*{password}" required>
<span th:if="${#fields.hasErrors('password')}" th:errors="*{password}"></span>
</div>
<div>
<label for="enabled">Enabled:</label>
<input type="checkbox" id="enabled" th:field="*{enabled}">
</div>
<div>
<button type="submit">Save</button>
</div>
</form>
</body>
</html>
```
完成以上步骤后,启动应用程序,访问"http://localhost:8080/users"即可查看用户列表,点击"Create New User"按钮即可添加新用户,点击"Edit"链接即可编辑用户信息,点击"Delete"链接即可删除用户信息。
以上就是一个简单的基于Spring Boot和Thymeleaf的电商后台应用程序,实现了注册信息表的CRUD功能。
阅读全文
相关推荐
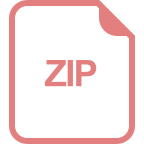
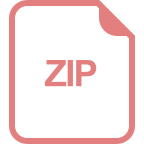
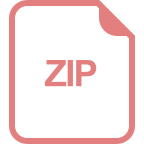
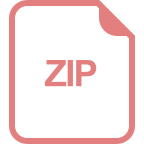
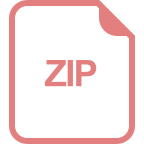
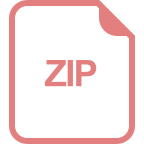
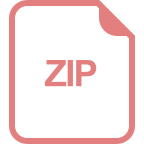
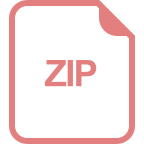
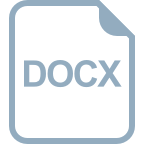
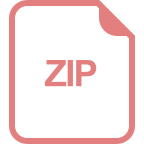
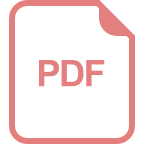