vue2 CKEditor5基本使用,图片上传,添加插件
时间: 2024-03-09 21:49:51 浏览: 17
首先,你需要安装 `@ckeditor/ckeditor5-vue2` 和 `@ckeditor/ckeditor5-build-classic` 两个依赖包。
```
npm install --save @ckeditor/ckeditor5-vue2 @ckeditor/ckeditor5-build-classic
```
然后在你的 Vue 组件中引入 CKEditor 组件:
```vue
<template>
<div>
<ckeditor :editor="editor" v-model="content" :config="editorConfig"></ckeditor>
</div>
</template>
<script>
import ClassicEditor from '@ckeditor/ckeditor5-build-classic'
import CKEditor from '@ckeditor/ckeditor5-vue2'
export default {
components: {
ckeditor: CKEditor.component
},
data() {
return {
content: '',
editorConfig: {
// 配置项
},
editor: ClassicEditor
}
}
}
</script>
```
在上面的代码中,我们引入了 `ClassicEditor`,它是一个预先配置好的编辑器,包含了常用的插件,如加粗、斜体、链接等。我们也可以自定义 `editorConfig`,来配置编辑器。
下面是一个常用的配置项示例:
```js
editorConfig: {
toolbar: {
items: [
'bold',
'italic',
'link',
'|',
'bulletedList',
'numberedList',
'|',
'imageUpload',
'blockQuote',
'insertTable',
'undo',
'redo'
]
},
image: {
toolbar: [
'imageTextAlternative',
'|',
'imageStyle:full',
'imageStyle:side',
'|',
'imageResize',
'|',
'imageUpload',
'imageUpload',
'imageUpload',
'imageUpload'
],
styles: [
'full',
'side'
]
},
language: 'zh-cn',
table: {
contentToolbar: [
'tableColumn',
'tableRow',
'mergeTableCells',
'tableCellProperties',
'tableProperties'
]
},
licenseKey: '',
simpleUpload: {
uploadUrl: '/your/upload/url',
headers: {
'X-CSRF-TOKEN': 'CSRF-Token'
}
}
}
```
上面的配置中,我们开启了图片上传功能,并且添加了表格插入、图片上传、撤销、重做等常用功能。同时也设置了语言为中文。
如果需要添加额外的插件,可以使用 `@ckeditor/ckeditor5-*` 的包名,比如添加字数统计插件:
```
npm install --save @ckeditor/ckeditor5-word-count
```
然后在 `editorConfig` 中添加:
```js
import WordCount from '@ckeditor/ckeditor5-word-count/src/wordcount';
editorConfig: {
plugins: [WordCount],
toolbar: [ 'wordCount' ]
}
```
这样就可以在编辑器中添加字数统计功能了。
我们也可以自定义上传图片的方法,需要在配置项中添加 `simpleUpload` 选项:
```js
editorConfig: {
simpleUpload: {
uploadUrl: '/your/upload/url',
headers: {
'X-CSRF-TOKEN': 'CSRF-Token'
},
// 自定义上传方法
async upload(file) {
// 这里写上传逻辑
const formData = new FormData();
formData.append('file', file);
const response = await axios.post('/your/upload/url', formData, {
headers: {
'Content-Type': 'multipart/form-data',
'X-CSRF-TOKEN': 'CSRF-Token'
}
});
return {
default: response.data.url
};
}
}
}
```
在上面的代码中,我们使用了 axios 发送了一个 `multipart/form-data` 的请求,然后返回上传的图片地址。
希望以上内容能够帮到你。
相关推荐
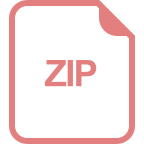
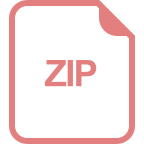














