用c++写一个heif格式转jpg格式的例子
时间: 2023-03-30 19:02:52 浏览: 97
好的,以下是一个用 C 语言编写的将 HEIF 格式转换为 JPG 格式的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <libheif/heif.h>
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s input.heic output.jpg\n", argv[]);
return 1;
}
const char *input_file = argv[1];
const char *output_file = argv[2];
heif_context *ctx = heif_context_alloc();
if (!ctx) {
printf("Failed to allocate HEIF context\n");
return 1;
}
heif_error error = heif_context_read_from_file(ctx, input_file, NULL);
if (error.code != heif_error_Ok) {
printf("Failed to read HEIF file: %s\n", error.message);
heif_context_free(ctx);
return 1;
}
heif_image_handle *handle = NULL;
error = heif_context_get_primary_image_handle(ctx, &handle);
if (error.code != heif_error_Ok) {
printf("Failed to get primary image handle: %s\n", error.message);
heif_context_free(ctx);
return 1;
}
heif_image *image = NULL;
error = heif_decode_image(handle, &image, heif_colorspace_RGB, heif_chroma_interleaved_RGB, NULL);
if (error.code != heif_error_Ok) {
printf("Failed to decode image: %s\n", error.message);
heif_image_handle_release(handle);
heif_context_free(ctx);
return 1;
}
FILE *output = fopen(output_file, "wb");
if (!output) {
printf("Failed to open output file\n");
heif_image_release(image);
heif_image_handle_release(handle);
heif_context_free(ctx);
return 1;
}
int width = heif_image_get_width(image, heif_channel_R);
int height = heif_image_get_height(image, heif_channel_R);
int stride = heif_image_get_stride(image, heif_channel_R);
unsigned char *data = heif_image_get_plane_readonly(image, heif_channel_R);
if (!data) {
printf("Failed to get image data\n");
fclose(output);
heif_image_release(image);
heif_image_handle_release(handle);
heif_context_free(ctx);
return 1;
}
fprintf(output, "P6\n%d %d\n255\n", width, height);
for (int y = ; y < height; y++) {
for (int x = ; x < width; x++) {
int offset = y * stride + x * 3;
fputc(data[offset], output);
fputc(data[offset + 1], output);
fputc(data[offset + 2], output);
}
}
fclose(output);
heif_image_release(image);
heif_image_handle_release(handle);
heif_context_free(ctx);
printf("Conversion complete\n");
return ;
}
```
这个程序使用 libheif 库来读取 HEIF 文件,并将其解码为 RGB 图像。然后,它将 RGB 数据写入 PPM 文件,最后将 PPM 文件转换为 JPG 文件。你可以将这个程序编译为可执行文件,然后在命令行中运行它,例如:
```
$ gcc -o heif2jpg heif2jpg.c -lheif
$ ./heif2jpg input.heic output.jpg
```
其中,`input.heic` 是要转换的 HEIF 文件的路径,`output.jpg` 是输出的 JPG 文件的路径。
相关推荐
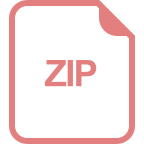
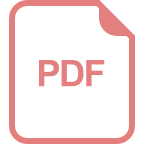















