c++ 读取文件第一行
时间: 2024-10-11 11:00:38 浏览: 38
在C++中,要读取文本文件的第一行,你可以使用`fstream`库,它是标准库的一部分,用于文件输入输出操作。以下是一个简单的例子:
```cpp
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("filename.txt"); // 替换 "filename.txt" 为你实际的文件名
if (inputFile.is_open()) { // 检查文件是否成功打开
std::string line;
getline(inputFile, line); // 读取一行直到遇到换行符
std::cout << "文件的第一行内容是: " << line << std::endl;
inputFile.close(); // 文件读完后记得关闭
} else {
std::cerr << "无法打开文件!" << std::endl;
}
return 0;
}
```
在这个示例中,`getline`函数用于从文件读取一行数据到`line`字符串中。如果文件打开失败,程序会打印错误信息。
相关问题
C++ 读取文件第二行
C++ 读取文件第二行有多种方法,以下是其中两种常用的方法:
1. 使用getline()函数读取文件的每一行,将其存储在一个字符串变量中,然后使用计数器变量来跟踪读取的行数,当计数器变量等于2时,输出该字符串变量即可。
2. 使用while循环和getline()函数读取文件的每一行,但只输出第二行,可以使用一个计数器变量来跟踪当前读取的行数,当计数器变量等于2时,输出该字符串变量即可。
示例代码如下:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
ifstream file("example.txt");
string line;
int count = 0;
while (getline(file, line)) {
count++;
if (count == 2) {
cout << line << endl;
break;
}
}
file.close();
return 0;
}
```
c++读取文件中的空格字符串
在C++中,可以使用fstream库读取文件。当读取空格字符串时,需要注意以下几点:
1. 使用getline函数读取一行字符串,而不是使用>>运算符读取单个单词。
2. 在读取时,需要指定空格为分隔符,即使用空格作为getline函数的第二个参数。
3. 如果要读取文件中的所有内容,需要使用while循环来重复读取。
以下是示例代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
ifstream myfile("example.txt"); //打开文件
string line;
while (getline(myfile, line, ' ')) { //按照空格分隔读取一行字符串
cout << line << endl;
}
myfile.close(); //关闭文件
return 0;
}
```
阅读全文
相关推荐
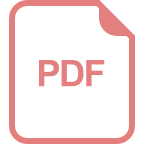
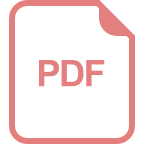
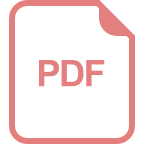
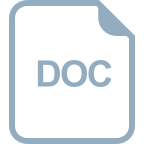
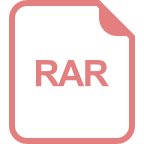
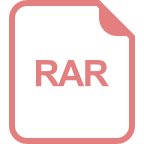
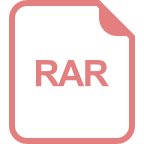
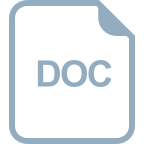
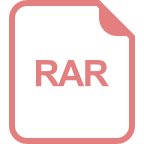
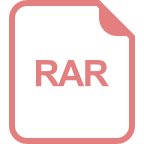
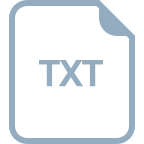
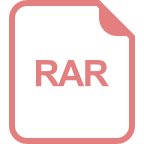
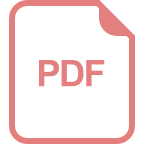
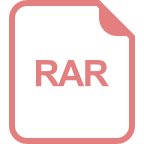
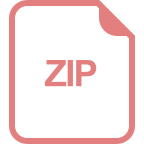

