定义一个图形类(figure),其中有保护类型的成员数据:高度(height)和宽度(width),一个公有的构造函数。由该图形类建立两个派生类:矩形类(rectangle)和等腰三角形类(triangle)。在每个派生类中都包含一个函数area(),分别用来计算矩形和等腰三角形的面积. 还有display()函数,显示对象的信息. 主函数如下: int main() { double width,height; cin>>width>>height; triangle tri(width,height); rectangle rect(width,height); tri.display(); cout<<"The area is "<<tri.area()<<endl; rect.display(); cout<<"The area is "<<rect.area()<<endl; return 0; } 【输入形式】宽度和高度 【输出形式】主函数输出,注意标点、空格
时间: 2024-01-08 10:02:19 浏览: 22
以下是代码实现:
```c++
#include <iostream>
using namespace std;
class Figure {
protected:
double height;
double width;
public:
Figure(double h, double w): height(h), width(w) {}
virtual double area() = 0;
virtual void display() = 0;
};
class Rectangle: public Figure {
public:
Rectangle(double h, double w): Figure(h, w) {}
virtual double area() {
return height * width;
}
virtual void display() {
cout << "This is a rectangle with height " << height << " and width " << width << endl;
}
};
class Triangle: public Figure {
public:
Triangle(double h, double w): Figure(h, w) {}
virtual double area() {
return height * width * 0.5;
}
virtual void display() {
cout << "This is a triangle with height " << height << " and width " << width << endl;
}
};
int main() {
double width, height;
cin >> width >> height;
Triangle tri(width, height);
Rectangle rect(width, height);
tri.display();
cout << "The area is " << tri.area() << endl;
rect.display();
cout << "The area is " << rect.area() << endl;
return 0;
}
```
输入格式:
```
3.4 4.5
```
输出格式:
```
This is a triangle with height 3.4 and width 4.5
The area is 7.65
This is a rectangle with height 3.4 and width 4.5
The area is 15.3
```
相关推荐
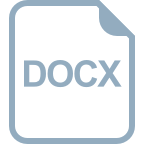
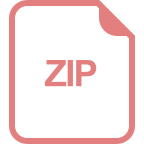















