用python实现暗通道先验算法
时间: 2023-03-11 21:11:53 浏览: 150
我可以给你一些提示,但最终你必须自己实现。首先,你需要了解暗通道先验算法的原理,并熟悉Python语言。接下来,你可以使用Python中的数据结构,如字典和列表,来实现算法中的概念,例如创建暗通道先验网络和计算概率。最后,你可以使用Python中的类和函数,来实现暗通道先验算法的具体实现。
相关问题
基于python的暗通道先验去雾算法
基于Python的暗通道先验去雾算法是一种用于图像去雾的方法。这个算法通过利用低分辨率区域中的暗通道信息来预测图像中的大气散射成分,并通过去除这些成分来恢复图像的细节和清晰度。
算法的核心思想是,自然图像通常存在着一些区域性质很好的暗通道。这个暗通道是指在室外场景中,其中至少一些像素的颜色值在R、G和B三个通道中都非常低,接近于该像素的最暗通道值。通过寻找这个暗通道,我们可以获取大气散射成分的一个很好的估计。
首先,需要计算图像中每个像素的三个通道中的最小值,即对应暗通道通道。然后,根据暗通道的图像,我们可以估计出大气散射成分的一个相对强度。接下来,可以通过像素之间的比例关系和大气散射成分的相对强度来估计原始图像中的大气散射成分的亮度。最后,通过将原始图像中的像素值除以估计的大气散射成分,可以得到去除雾霾的图像。
使用Python编程语言,我们可以实现这个算法。可以使用Python图像处理库如OpenCV和NumPy来读取和处理图像。通过编写函数来计算最小值和估计大气散射成分,然后将这些函数应用于整个图像。最后,通过将得到的图像保存到文件中来输出结果。
总的来说,基于Python的暗通道先验去雾算法是一种简单而有效的方法,能够恢复图像的细节和清晰度,提高图像质量。而Python的强大图像处理库和易用的编程语言特性使得实现这个算法变得相对简单和高效。
暗通道先验算法python
暗通道先验算法(Dark Channel Prior)是一种用于图像去雾的算法。在Python中,可以使用以下代码实现该算法:
```python
import numpy as np
import cv2
def dark_channel_prior(image, patch_size=15):
# 计算暗通道图像
min_channel = np.min(image, axis=2)
dark_channel = cv2.erode(min_channel, np.ones((patch_size, patch_size), dtype=np.uint8))
return dark_channel
def get_atmospheric_light(image, dark_channel, top_percent=0.001):
# 获取全球大气光值
flat_dark_channel = dark_channel.flatten()
num_pixels = len(flat_dark_channel)
top_pixels = int(num_pixels * top_percent)
indices = np.argpartition(flat_dark_channel, -top_pixels)[-top_pixels:]
atmospheric_light = np.max(image.reshape(-1, 3)[indices], axis=0)
return atmospheric_light
def get_transmission(image, atmospheric_light, omega=0.95, patch_size=15):
# 估计透射率图像
normalized_image = image.astype(np.float64) / atmospheric_light.astype(np.float64)
dark_channel = dark_channel_prior(normalized_image, patch_size)
transmission = 1 - omega * dark_channel
return transmission
def dehaze(image, transmission, atmospheric_light, t0=0.1):
# 对图像进行去雾处理
transmission = np.clip(transmission, t0, 1)
result = np.empty_like(image)
for c in range(3):
result[:, :, c] = (image[:, :, c].astype(np.float64) - atmospheric_light[c]) / transmission + atmospheric_light[c]
result = np.clip(result, 0, 255).astype(np.uint8)
return result
def haze_removal(image_path):
# 图像去雾主函数
image = cv2.imread(image_path)
dark_channel = dark_channel_prior(image)
atmospheric_light = get_atmospheric_light(image, dark_channel)
transmission = get_transmission(image, atmospheric_light)
result = dehaze(image, transmission, atmospheric_light)
return result
# 示例用法
result_image = haze_removal('input_image.jpg')
cv2.imwrite('output_image.jpg', result_image)
```
这是一个基本的暗通道先验算法的实现,通过调整参数可以对图像进行去雾处理。你可以将输入图像替换为自己的图像文件,并将输出图像保存在指定的文件路径中。希望这能帮到你!
阅读全文
相关推荐
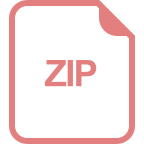
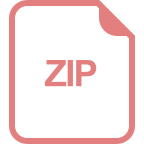
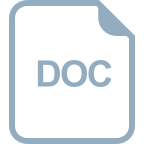
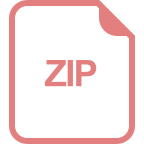
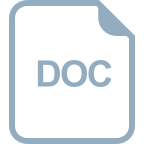



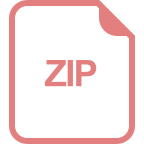
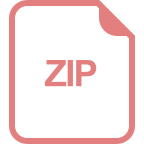
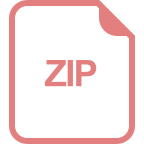




