用C语言写一个生产者与消费者的demo
时间: 2024-05-14 09:14:24 浏览: 95
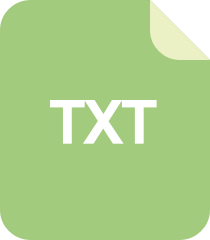
生产着与消费者者 c语言
这里是一个基于Linux的C语言生产者与消费者的demo,使用了线程和信号量:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE];
int in = 0;
int out = 0;
sem_t empty;
sem_t full;
pthread_mutex_t mutex;
void *producer(void *arg) {
int item;
while (1) {
item = rand() % 1000; // 生成一个随机的生产者元素
sem_wait(&empty); // 等待空缓冲区
pthread_mutex_lock(&mutex); // 加锁
buffer[in] = item; // 放入缓冲区
printf("Producer produced %d\n", item);
in = (in + 1) % BUFFER_SIZE; // 更新缓冲区索引
pthread_mutex_unlock(&mutex); // 解锁
sem_post(&full); // 发送满信号
}
}
void *consumer(void *arg) {
int item;
while (1) {
sem_wait(&full); // 等待满缓冲区
pthread_mutex_lock(&mutex); // 加锁
item = buffer[out]; // 取出缓冲区元素
printf("Consumer consumed %d\n", item);
out = (out + 1) % BUFFER_SIZE; // 更新缓冲区索引
pthread_mutex_unlock(&mutex); // 解锁
sem_post(&empty); // 发送空信号
}
}
int main() {
sem_init(&empty, 0, BUFFER_SIZE); // 初始化空信号量为BUFFER_SIZE
sem_init(&full, 0, 0); // 初始化满信号量为0
pthread_mutex_init(&mutex, NULL); // 初始化锁
pthread_t producer_thread, consumer_thread;
pthread_create(&producer_thread, NULL, producer, NULL); // 创建生产者线程
pthread_create(&consumer_thread, NULL, consumer, NULL); // 创建消费者线程
pthread_join(producer_thread, NULL); // 等待生产者线程结束
pthread_join(consumer_thread, NULL); // 等待消费者线程结束
pthread_mutex_destroy(&mutex); // 销毁锁
sem_destroy(&empty); // 销毁空信号量
sem_destroy(&full); // 销毁满信号量
return 0;
}
```
在Linux系统上使用以下命令进行编译和运行:
```
gcc -o demo demo.c -lpthread -lrt
./demo
```
阅读全文
相关推荐
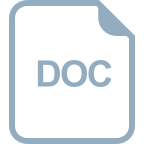
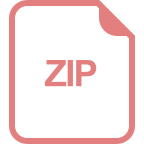
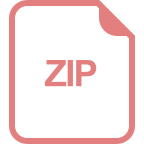
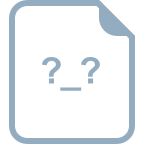
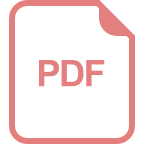
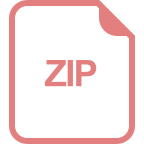
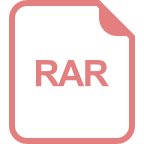
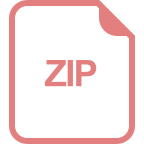
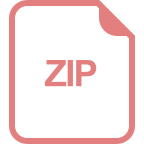
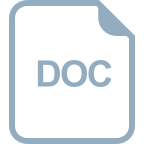
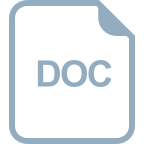
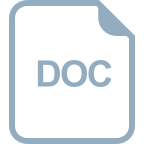
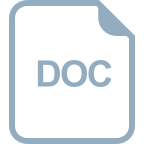
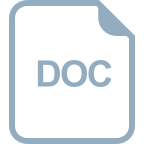
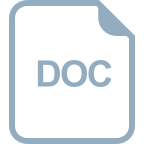
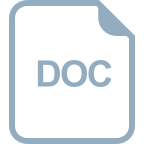