请帮我写一段python利用smote律平衡数据集的代码
时间: 2023-06-04 09:05:48 浏览: 150
好的,以下是一个使用SMOTE算法实现数据集平衡的Python代码示例:
```python
from imblearn.over_sampling import SMOTE
import numpy as np
import pandas as pd
# 加载不平衡的数据集
X = pd.read_csv("unbalanced_data.csv")
# 提取目标变量
y = X.pop("target")
# 实例化SMOTE算法
smote = SMOTE(random_state=42)
# 使用SMOTE算法平衡数据集
X_resampled, y_resampled = smote.fit_resample(X, y)
# 打印新的平衡数据集的形状
print("样本数: ", len(X_resampled))
print("正样本数: ", np.count_nonzero(y_resampled == 1))
print("负样本数: ", np.count_nonzero(y_resampled == 0))
```
以上代码通过使用SMOTE算法平衡了原始数据集,并将平衡后的数据集存储在`X_resampled`和`y_resampled`变量中。如果你需要更多关于这个代码的帮助,请随时告诉我!
相关问题
写出一段利用smote算法处理不平衡图像数据集的代码示例
以下是利用SMOTE算法处理不平衡图像数据集的代码示例:
```python
from imblearn.over_sampling import SMOTE
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
# 生成不平衡的样本数据集
X, y = make_classification(n_classes=2, class_sep=2,
weights=[0.1, 0.9], n_informative=3,
n_redundant=1, flip_y=0, n_features=20,
n_clusters_per_class=1, n_samples=1000, random_state=10)
# 将数据集划分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=10)
# 对不平衡数据进行SMOTE处理
smote = SMOTE(random_state=10)
X_train_smote, y_train_smote = smote.fit_sample(X_train, y_train)
# 输出SMOTE处理后的样本数量
print("SMOTE处理后的样本数量:", len(X_train_smote))
```
在上面的代码中,我们首先使用`make_classification`函数生成了一个不平衡的样本数据集。然后,我们使用`train_test_split`函数将数据集划分为训练集和测试集。接着,我们使用`SMOTE`类进行SMOTE处理,得到了新的训练集和标签。最后,我们输出了SMOTE处理后的样本数量。
需要注意的是,上面的代码只是对不平衡样本进行了SMOTE处理,如果需要进行模型训练和测试,还需要进一步处理数据集。
利用特征熵结合SMOTE过采样写一段python代码
### 回答1:
from imblearn.over_sampling import SMOTE
from sklearn.feature_selection import mutual_info_classif# select feature
X = data.iloc[:, :-1]
y = data.iloc[:, -1]# calculate mutual information
mi = mutual_info_classif(X, y)# select features with highest entropy
mi_best = np.argsort(mi)[-5:]
X_best = X.iloc[:, mi_best]# over-sampling
sm = SMOTE(random_state=42)
X_resampled, y_resampled = sm.fit_resample(X_best, y)
### 回答2:
利用特征熵结合SMOTE(Synthetic Minority Over-sampling Technique)过采样可以有效处理不平衡数据集的问题。下面是一个简单的Python代码示例:
```python
import pandas as pd
import numpy as np
from imblearn.over_sampling import SMOTE
from sklearn.feature_selection import SelectKBest
from sklearn.feature_selection import mutual_info_classif
def oversampling_with_feature_entropy(X, y, k):
# 计算特征的熵
selector = SelectKBest(score_func=mutual_info_classif, k=k)
X_selected = selector.fit_transform(X, y)
# 使用SMOTE过采样
oversampler = SMOTE()
X_oversampled, y_oversampled = oversampler.fit_resample(X_selected, y)
return X_oversampled, y_oversampled
# 读取数据集
data = pd.read_csv("data.csv")
X = data.iloc[:, :-1]
y = data.iloc[:, -1]
# 使用特征熵结合SMOTE过采样
X_oversampled, y_oversampled = oversampling_with_feature_entropy(X, y, k=5)
# 打印过采样后的数据集
print("Over-sampled dataset:")
print(pd.concat([pd.DataFrame(X_oversampled), pd.DataFrame(y_oversampled)], axis=1))
```
以上代码中,我们首先利用`SelectKBest`函数计算特征的熵,然后保留熵最高的k个特征。接下来,使用`SMOTE`类对经过特征选择后的数据集进行过采样。最后,将过采样后的数据集与对应的标签合并并打印出来。
注意,以上代码中使用了`imblearn`库中的`SMOTE`类进行过采样操作,因此需要事先安装该库。
### 回答3:
特征熵是一种用于度量特征在样本集中的变化程度的指标,而SMOTE(合成少数类过采样技术)是一种用于处理类别不平衡问题的方法。在给定一个数据集时,可以将特征熵与SMOTE结合使用来增加数据集中少数类的样本,以达到数据平衡的目的。
下面是一段使用特征熵结合SMOTE过采样的Python代码:
```python
import numpy as np
from collections import Counter
from sklearn.datasets import make_classification
from sklearn.feature_selection import SelectKBest, mutual_info_classif
from imblearn.over_sampling import SMOTE
# 生成一个样本不平衡的数据集
X, y = make_classification(n_samples=1000, n_features=20, n_informative=2, n_redundant=18, weights=[0.9, 0.1])
# 计算特征熵
selector = SelectKBest(score_func=mutual_info_classif, k=10)
X_selected = selector.fit_transform(X, y)
# 查看特征熵的结果
print("特征熵:")
print(selector.scores_)
# 使用SMOTE进行过采样
smote = SMOTE()
X_resampled, y_resampled = smote.fit_resample(X_selected, y)
# 查看过采样后的样本分布
print("过采样后的样本分布:")
print(Counter(y_resampled))
```
在这段代码中,首先使用`make_classification`函数生成一个样本不平衡的数据集。然后使用`SelectKBest`和`mutual_info_classif`计算特征熵,选择前10个最具相关性的特征。接下来,使用`SMOTE`进行过采样,生成平衡后的数据集。最后,使用`Counter`统计过采样后的样本分布情况,以验证过采样的效果。
需要注意的是,这里使用了`sklearn`库中的`make_classification`、`SelectKBest`、`mutual_info_classif`等函数,以及`imblearn`库中的`SMOTE`类。在运行代码之前,需要确保已经安装了这些库,并且导入相应的模块。
相关推荐
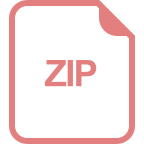











