unzip 压缩及解压文件夹c++示例
时间: 2023-10-11 08:08:15 浏览: 109
下面是一个使用C++的例子,展示如何压缩和解压文件夹:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <cstring>
#include "zip.h"
#include "unzip.h"
void zipFolder(const std::string& folderPath, const std::string& zipPath) {
zipFile zf = zipOpen(zipPath.c_str(), APPEND_STATUS_CREATE);
if (zf == nullptr) {
std::cerr << "Error opening " << zipPath << " for writing!" << std::endl;
return;
}
std::vector<char> buffer(1024 * 1024);
dirent* entry;
DIR* dir = opendir(folderPath.c_str());
while ((entry = readdir(dir))) {
if (entry->d_type == DT_REG) {
std::string filePath = folderPath + "/" + entry->d_name;
std::ifstream file(filePath, std::ios::binary);
if (!file) {
std::cerr << "Error opening " << filePath << " for reading!" << std::endl;
continue;
}
zip_fileinfo fileInfo = {0};
zipOpenNewFileInZip(zf, entry->d_name, &fileInfo, nullptr, 0, nullptr, 0, nullptr, Z_DEFLATED, Z_DEFAULT_COMPRESSION);
while (file.read(buffer.data(), buffer.size())) {
zipWriteInFileInZip(zf, buffer.data(), static_cast<unsigned int>(file.gcount()));
}
zipCloseFileInZip(zf);
}
}
closedir(dir);
zipClose(zf, nullptr);
}
void unzipFolder(const std::string& zipPath, const std::string& folderPath) {
unzFile uf = unzOpen(zipPath.c_str());
if (uf == nullptr) {
std::cerr << "Error opening " << zipPath << " for reading!" << std::endl;
return;
}
std::vector<char> buffer(1024 * 1024);
unz_global_info64 gi;
unzGetGlobalInfo64(uf, &gi);
for (uLong i = 0; i < gi.number_entry; ++i) {
unz_file_info64 fileInfo;
char fileName[MAX_PATH];
unzGetCurrentFileInfo64(uf, &fileInfo, fileName, MAX_PATH, nullptr, 0, nullptr, 0);
unzOpenCurrentFile(uf);
std::string filePath = folderPath + "/" + std::string(fileName);
std::ofstream file(filePath, std::ios::binary);
if (!file) {
std::cerr << "Error opening " << filePath << " for writing!" << std::endl;
continue;
}
while (true) {
int bytesRead = unzReadCurrentFile(uf, buffer.data(), buffer.size());
if (bytesRead < 0) {
std::cerr << "Error reading " << fileName << " from zip file!" << std::endl;
break;
}
if (bytesRead == 0) {
break;
}
file.write(buffer.data(), bytesRead);
}
file.close();
unzCloseCurrentFile(uf);
unzGoToNextFile(uf);
}
unzClose(uf);
}
int main() {
std::string folderPath = "/path/to/folder";
std::string zipPath = "/path/to/archive.zip";
zipFolder(folderPath, zipPath);
std::string unzipFolderPath = "/path/to/unzip/folder";
unzipFolder(zipPath, unzipFolderPath);
return 0;
}
```
这个例子使用了zlib库来进行压缩和解压缩,需要在代码中包含"zip.h"和"unzip.h"头文件,并且需要在编译时链接zlib库。
阅读全文
相关推荐
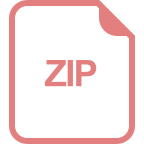
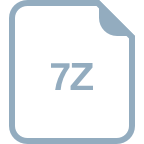
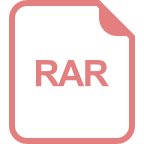
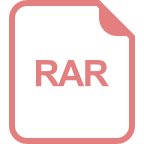
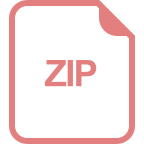
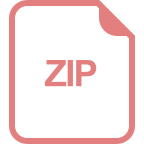
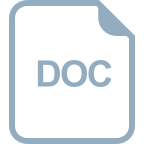
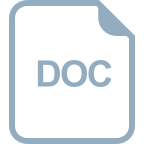
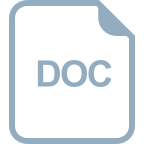


