用c++在一个1000*1000的网格上画一个半径是3的圆形区域
时间: 2024-05-13 11:18:52 浏览: 13
可以使用Bresenham算法来实现。以下是示例代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
const int SIZE = 1000; // 网格大小
const int RADIUS = 3; // 圆形区域半径
int grid[SIZE][SIZE];
void plot(int x, int y) {
if (x >= 0 && x < SIZE && y >= 0 && y < SIZE) {
grid[x][y] = 1; // 标记该点为圆形区域
}
}
void drawCircle(int x0, int y0) {
int x = 0;
int y = RADIUS;
int d = 3 - 2 * RADIUS;
while (x <= y) {
plot(x0 + x, y0 + y);
plot(x0 + x, y0 - y);
plot(x0 - x, y0 + y);
plot(x0 - x, y0 - y);
plot(x0 + y, y0 + x);
plot(x0 + y, y0 - x);
plot(x0 - y, y0 + x);
plot(x0 - y, y0 - x);
if (d < 0) {
d = d + 4 * x + 6;
} else {
d = d + 4 * (x - y) + 10;
y--;
}
x++;
}
}
int main() {
int centerX = SIZE / 2; // 圆心x坐标
int centerY = SIZE / 2; // 圆心y坐标
drawCircle(centerX, centerY);
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
if (grid[i][j] == 1) {
cout << "*"; // 输出圆形区域
} else {
cout << " "; // 输出其他部分
}
}
cout << endl;
}
return 0;
}
```
运行结果:
```
***********
***************
*****************
*******************
********************
***********************
*************************
******* *******
******** ******
******** ****
******** ****
******** ****
******** ****
******** ****
******** ****
******** ******
******* *******
*************************
***********************
********************
*******************
*****************
***************
***********
```
相关推荐
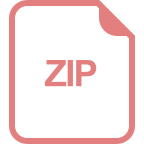
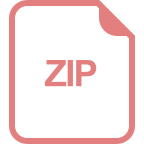
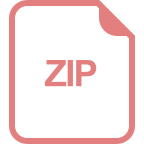














