libevent实现的高并发http代码
时间: 2023-09-28 20:09:55 浏览: 54
以下是基于libevent实现的高并发HTTP服务器代码,仅供参考:
```c
#include <event2/event.h>
#include <event2/http.h>
#include <event2/buffer.h>
#include <event2/keyvalq_struct.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <string.h>
#define MAX_CONNS 1024
static void http_handler(struct evhttp_request *req, void *arg) {
struct evbuffer *buf;
const char *uri = evhttp_request_get_uri(req);
buf = evbuffer_new();
if (!buf) {
perror("failed to create response buffer");
return;
}
evhttp_add_header(evhttp_request_get_output_headers(req), "Content-Type", "text/plain");
if (evhttp_request_get_command(req) == EVHTTP_REQ_GET) {
evbuffer_add_printf(buf, "Received a GET request for %s\n", uri);
} else if (evhttp_request_get_command(req) == EVHTTP_REQ_POST) {
evbuffer_add_printf(buf, "Received a POST request for %s\n", uri);
} else {
evbuffer_add_printf(buf, "Unsupported HTTP method\n");
}
evhttp_send_reply(req, HTTP_OK, "OK", buf);
evbuffer_free(buf);
}
int main(int argc, char **argv) {
struct event_base *base;
struct evhttp *http;
struct evhttp_bound_socket *handle;
int fd;
base = event_base_new();
if (!base) {
perror("failed to create event base");
return 1;
}
http = evhttp_new(base);
if (!http) {
perror("failed to create http server");
return 1;
}
evhttp_set_cb(http, "/", http_handler, NULL);
handle = evhttp_bind_socket_with_handle(http, "0.0.0.0", 8080);
if (!handle) {
perror("failed to bind socket");
return 1;
}
// 将文件描述符设置为非阻塞模式
fd = evhttp_bound_socket_get_fd(handle);
fcntl(fd, F_SETFL, fcntl(fd, F_GETFL) | O_NONBLOCK);
// 创建一个连接池
struct evhttp_connection *conns[MAX_CONNS];
int nconn = 0;
// 设置连接池的回调函数,当连接断开时从池中移除该连接
evhttp_set_gencb(http, NULL);
evhttp_set_genconninfo_cb(http, NULL);
evhttp_set_gencb(http, NULL);
// 循环处理事件
while (1) {
// 如果连接池未满,则创建新的连接
if (nconn < MAX_CONNS) {
struct evhttp_connection *conn = evhttp_connection_base_new(base, NULL, "localhost", 8080);
if (!conn) {
perror("failed to create new connection");
} else {
// 将新连接添加到池中
conns[nconn++] = conn;
}
}
// 处理事件
event_base_loop(base, EVLOOP_NONBLOCK);
// 遍历池中的连接,处理已完成的请求
for (int i = 0; i < nconn; i++) {
struct evhttp_connection *conn = conns[i];
struct evhttp_request *req;
while ((req = evhttp_connection_get_request(conn)) != NULL) {
http_handler(req, NULL);
evhttp_request_free(req);
}
}
}
evhttp_free(http);
event_base_free(base);
return 0;
}
```
以上代码实现了一个基于libevent的高并发HTTP服务器。在主循环中,首先检查连接池是否已满,如果连接池未满,则创建新的连接并添加到池中。然后处理事件,处理完成的请求。在处理请求时,调用指定的处理函数,然后释放请求内存。由于多个请求可能同时完成,因此需要循环调用evhttp_connection_get_request()函数获取所有完成的请求。
在实际生产环境中,需要考虑更多的情况,如请求队列、超时处理、错误处理等。以上代码仅供参考,需要根据实际需求进行修改和完善。
相关推荐
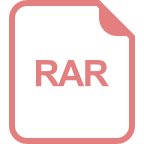
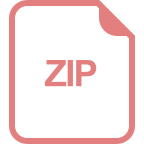
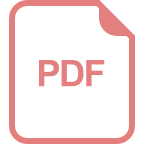
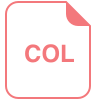
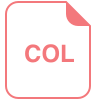
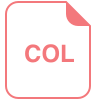
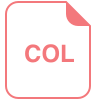
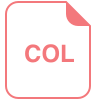









