unordered_map.find用法
时间: 2023-10-12 08:14:34 浏览: 271
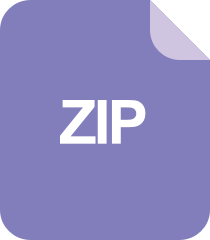
IATHook的代码 unorder-map管理
unordered_map.find() 是 C++ STL 中 unordered_map 容器提供的一个函数,用于在 unordered_map 中查找指定键的迭代器。例如,以下是 unordered_map.find() 的使用方法:
```
#include <iostream>
#include <unordered_map>
using namespace std;
int main()
{
unordered_map<string, int> mymap = {{"foo", 1}, {"bar", 2}, {"baz", 3}};
// 查找键为 "foo" 的元素
auto it = mymap.find("foo");
if (it != mymap.end()) {
cout << "Key found: " << it->first << ", value is " << it->second << endl;
} else {
cout << "Key not found." << endl;
}
return 0;
}
```
输出:
```
Key found: foo, value is 1
```
如果找到了指定键的元素,则返回指向该元素的迭代器。如果没有找到,则返回 unordered_map::end() 迭代器。
阅读全文
相关推荐
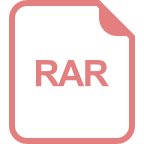











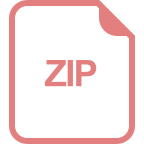



