写一段cordic算法的c语言代码
时间: 2024-05-05 14:16:49 浏览: 16
以下是一个简单的CORDIC算法的C语言代码示例:
```c
#include <stdio.h>
#include <math.h>
#define N 16
void cordic_rotation(double *x, double *y, double *z, int n) {
double tx, ty, tz;
for (int i = 0; i < n; i++) {
tz = *z;
if (*z < 0) {
tx = *x + (*y >> i);
ty = *y - (*x >> i);
*x = tx;
*y = ty;
*z += atan(pow(2, -i));
} else {
tx = *x - (*y >> i);
ty = *y + (*x >> i);
*x = tx;
*y = ty;
*z -= atan(pow(2, -i));
}
}
}
int main() {
double x = 0.75, y = 0.25, z = atan(y/x);
cordic_rotation(&x, &y, &z, N);
printf("x = %f, y = %f, z = %f\n", x, y, z);
return 0;
}
```
在这个示例中,我们使用CORDIC算法来计算给定初始值(x, y)的极坐标(r, theta),其中r和theta定义如下:
r = sqrt(x^2 + y^2)
theta = atan(y / x)
我们使用CORDIC算法来计算theta的值,它被存储在z变量中。我们使用16位迭代来获得更高的精度,但这个数字可以根据需要更改。最后,我们输出计算出的(x, y, z)值。
相关推荐










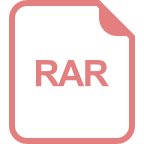