用HTML写一个杯子中放出烟花的代码
时间: 2024-05-16 11:18:21 浏览: 165
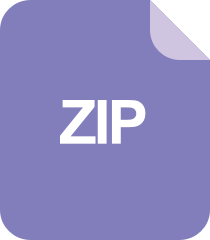
跨年烟花代码html效果

抱歉,HTML本身无法实现动态效果,需要使用JavaScript或其他前端框架来实现。以下是一个基于JavaScript的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>烟花杯</title>
<style>
body {
background-color: black;
}
canvas {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script>
const canvas = document.getElementById('canvas');
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
const ctx = canvas.getContext('2d');
class Firework {
constructor(x, y, color) {
this.x = x;
this.y = y;
this.color = color;
this.particles = [];
for (let i = 0; i < 20; i++) {
this.particles.push(new Particle(this.x, this.y, this.color));
}
}
update() {
for (let i = 0; i < this.particles.length; i++) {
this.particles[i].update();
if (this.particles[i].done()) {
this.particles.splice(i, 1);
}
}
}
draw() {
for (let i = 0; i < this.particles.length; i++) {
this.particles[i].draw();
}
}
}
class Particle {
constructor(x, y, color) {
this.x = x;
this.y = y;
this.color = color;
this.vx = Math.random() * 10 - 5;
this.vy = Math.random() * 10 - 5;
this.gravity = 0.2;
this.alpha = 1;
}
update() {
this.x += this.vx;
this.y += this.vy;
this.vy += this.gravity;
this.alpha -= 0.01;
}
done() {
return this.alpha <= 0;
}
draw() {
ctx.fillStyle = this.color;
ctx.globalAlpha = this.alpha;
ctx.beginPath();
ctx.arc(this.x, this.y, 5, 0, 2 * Math.PI);
ctx.fill();
}
}
const fireworks = [];
function animate() {
requestAnimationFrame(animate);
ctx.fillStyle = 'rgba(0, 0, 0, 0.1)';
ctx.fillRect(0, 0, canvas.width, canvas.height);
for (let i = 0; i < fireworks.length; i++) {
fireworks[i].update();
fireworks[i].draw();
}
}
canvas.addEventListener('click', function(event) {
const color = 'hsl(' + Math.random() * 360 + ', 100%, 50%)';
fireworks.push(new Firework(event.clientX, event.clientY, color));
});
animate();
</script>
</body>
</html>
```
你可以将该代码保存为`fireworks.html`文件,然后在浏览器中打开该文件,点击页面的任意位置即可看到“烟花杯”的效果。
阅读全文
相关推荐
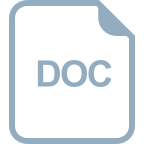
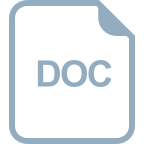
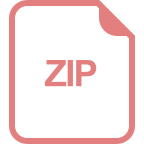
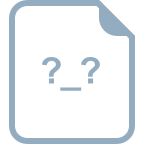
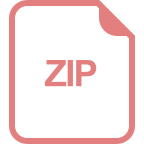
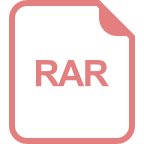


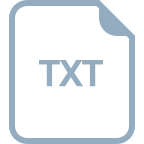
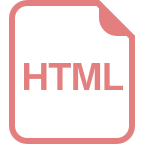
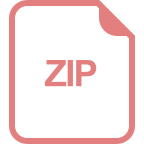
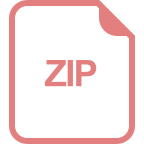
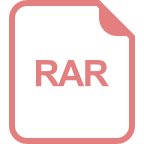