JavaFX画出汽车雨刮器,并且可以进行升档降档来调节速度
时间: 2023-07-16 15:13:50 浏览: 157
下面是一个简单的JavaFX程序,用于绘制汽车雨刮器和调节速度:
```java
import javafx.animation.AnimationTimer;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class CarWindshieldWiper extends Application {
private static final int WIDTH = 400;
private static final int HEIGHT = 400;
private static final int WIPER_SPEED = 10;
private static final int MAX_SPEED = 5;
private int currentSpeed = 1;
private boolean isGoingUp = true;
@Override
public void start(Stage primaryStage) {
Canvas canvas = new Canvas(WIDTH, HEIGHT);
GraphicsContext gc = canvas.getGraphicsContext2D();
AnimationTimer timer = new AnimationTimer() {
long lastUpdate = 0;
@Override
public void handle(long now) {
if (lastUpdate != 0) {
long elapsedNanoSeconds = now - lastUpdate;
double elapsedSeconds = elapsedNanoSeconds / 1_000_000_000.0;
gc.clearRect(0, 0, WIDTH, HEIGHT);
drawWindshield(gc);
updateWiperPosition(elapsedSeconds);
drawWiper(gc);
}
lastUpdate = now;
}
};
timer.start();
StackPane root = new StackPane(canvas);
Scene scene = new Scene(root);
scene.setOnKeyPressed(event -> {
switch (event.getCode()) {
case UP:
if (currentSpeed < MAX_SPEED) {
currentSpeed++;
}
break;
case DOWN:
if (currentSpeed > 1) {
currentSpeed--;
}
break;
default:
break;
}
});
primaryStage.setScene(scene);
primaryStage.show();
}
private void drawWindshield(GraphicsContext gc) {
gc.setFill(Color.BLUE);
gc.fillRect(50, 50, 300, 200);
gc.setFill(Color.GRAY);
gc.fillOval(100, 100, 200, 100);
}
private void updateWiperPosition(double elapsedSeconds) {
double deltaPosition = currentSpeed * WIPER_SPEED * elapsedSeconds;
if (isGoingUp) {
Wiper.getInstance().moveUp(deltaPosition);
} else {
Wiper.getInstance().moveDown(deltaPosition);
}
if (Wiper.getInstance().getY() < 75) {
isGoingUp = false;
} else if (Wiper.getInstance().getY() > 225) {
isGoingUp = true;
}
}
private void drawWiper(GraphicsContext gc) {
gc.setFill(Color.WHITE);
gc.fillRect(Wiper.getInstance().getX(), Wiper.getInstance().getY(), 75, 5);
}
public static void main(String[] args) {
launch(args);
}
private static class Wiper {
private static final Wiper INSTANCE = new Wiper();
private double x = 100;
private double y = 150;
private Wiper() {}
public static Wiper getInstance() {
return INSTANCE;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
public void moveUp(double delta) {
y -= delta;
}
public void moveDown(double delta) {
y += delta;
}
}
}
```
这个程序使用JavaFX的Canvas绘制汽车雨刮器和雨刮器的位置。程序使用AnimationTimer类来更新雨刮器的位置,并使用按键事件来调整速度。Wiper类是一个单例,用于跟踪雨刮器的位置。
阅读全文
相关推荐
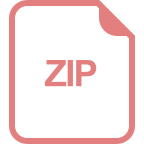
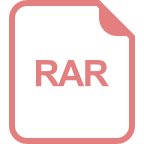
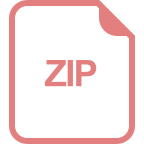
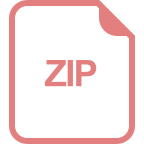
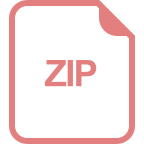
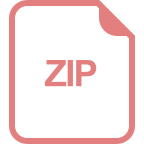
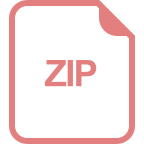
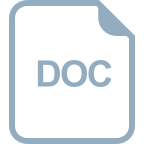
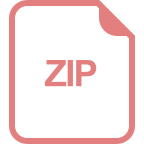
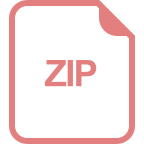
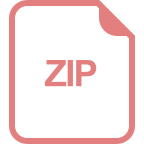
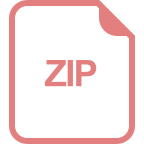
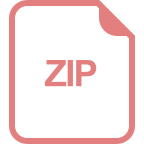
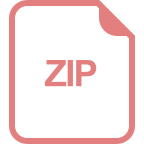
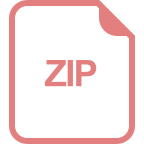
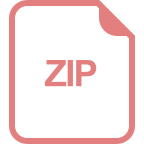
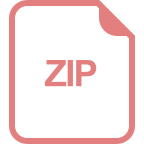