优化Timer timer = new Timer(); timer.schedule(new TimerTask() { public void run() { cells = GameService.generate(cells); Time.setText(" " + generation); ++generation; for (int i = 0; i < row; i++) for (int j = 0; j < col; j++) { if (cells.getCell(i, j) == CellState.LIVE.getValue()) btns[i][j].setBackground(Color.black); else btns[i][j].setBackground(Color.white); } } }, 0, 1000);//更改每次迭代时间 此处设为每一秒迭代一次 }
时间: 2024-03-24 14:40:55 浏览: 24
在这段代码中,使用了Java自带的Timer和TimerTask类来实现定时执行任务。但是,这种方式有一些缺点:
1. Timer是单线程执行任务的,如果任务执行时间较长,会导致后续任务的延迟执行。
2. 如果TimerTask中的任务执行出现异常,Timer会停止执行所有任务。
为了避免这些问题,可以考虑使用Java的ScheduledExecutorService类来代替Timer。
下面是使用ScheduledExecutorService优化后的代码:
```java
ScheduledExecutorService scheduledExecutorService = Executors.newSingleThreadScheduledExecutor();
scheduledExecutorService.scheduleAtFixedRate(new Runnable() {
@Override
public void run() {
cells = GameService.generate(cells);
Time.setText(" " + generation);
++generation;
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
if (cells.getCell(i, j) == CellState.LIVE.getValue()) {
btns[i][j].setBackground(Color.black);
} else {
btns[i][j].setBackground(Color.white);
}
}
}
}
}, 0, 1000, TimeUnit.MILLISECONDS);
```
这里使用了ScheduledExecutorService的scheduleAtFixedRate方法来定时执行任务,可以指定任务首次执行的延迟时间和每次执行的间隔时间。此外,ScheduledExecutorService是多线程执行的,可以避免上述问题。
相关推荐
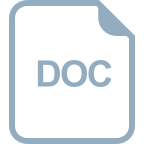
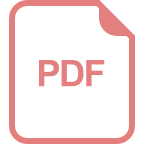














