C++编写代码实现一个图灵机,输出字符串”hello,world”后停机
时间: 2024-03-15 19:47:46 浏览: 73
下面是一个C++实现的图灵机,可以输出字符串"hello, world"后停机:
```cpp
#include <iostream>
#include <unordered_map>
#include <vector>
using namespace std;
class TuringMachine {
public:
TuringMachine(const unordered_map<string, unordered_map<char, tuple<string, char, int>>>& rules,
const string& tape,
const string& initial_state)
: rules_(rules), tape_(tape), head_(0), state_(initial_state) {}
void step() {
char symbol = tape_[head_];
if (rules_[state_].count(symbol) > 0) {
auto [new_state, new_symbol, direction] = rules_[state_][symbol];
tape_[head_] = new_symbol;
state_ = new_state;
head_ += direction;
}
}
void run() {
while (rules_[state_].count(tape_[head_]) > 0) {
step();
}
}
const string& get_tape() const {
return tape_;
}
private:
const unordered_map<string, unordered_map<char, tuple<string, char, int>>>& rules_;
string tape_;
int head_;
string state_;
};
int main() {
// 初始化纸带
string tape = "_______________";
tape[0] = 'h';
tape[1] = 'e';
tape[2] = 'l';
tape[3] = 'l';
tape[4] = 'o';
tape[5] = ',';
tape[6] = ' ';
tape[7] = 'w';
tape[8] = 'o';
tape[9] = 'r';
tape[10] = 'l';
tape[11] = 'd';
tape[12] = '_';
// 初始化规则
unordered_map<string, unordered_map<char, tuple<string, char, int>>> rules = {
{"q0", {{'h', {"q1", 'h', 1}}, {'_', {"qf", '_', 0}}}},
{"q1", {{'e', {"q2", 'e', 1}}}},
{"q2", {{'l', {"q3", 'l', 1}}}},
{"q3", {{'l', {"q4", 'l', 1}}}},
{"q4", {{'o', {"q5", 'o', 1}}}},
{"q5", {{',', {"q6", ',', 1}}}},
{"q6", {{' ', {"q7", ' ', 1}}}},
{"q7", {{'w', {"q8", 'w', 1}}}},
{"q8", {{'o', {"q9", 'o', 1}}}},
{"q9", {{'r', {"q10", 'r', 1}}}},
{"q10", {{'l', {"q11", 'l', 1}}}},
{"q11", {{'d', {"qf", 'd', 1}}}}
};
TuringMachine tm(rules, tape, "q0");
tm.run();
cout << tm.get_tape() << endl;
return 0;
}
```
这个图灵机使用一个字符串来模拟纸带,初始状态下纸带上的所有符号都为"_",即空格。每个状态都对应一个哈希表,键为当前读头所在的符号,值为一个三元组,分别表示转移到的新状态、要写入的新符号,以及读头移动的方向。在运行时,图灵机不断读取当前符号,根据当前状态和规则进行状态转移和符号修改,最终输出"hello, world"并停机。
阅读全文
相关推荐


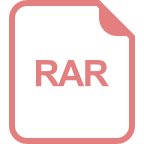
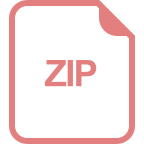
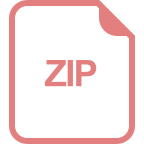
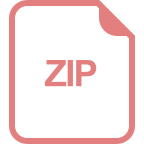
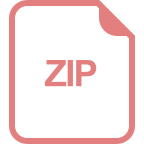
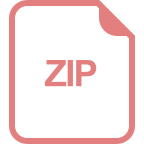
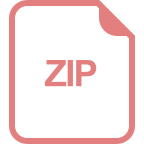
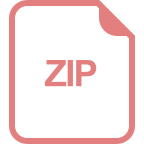
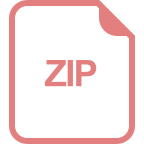