import numpy as np import sys from torchvision import transforms sys.path.append("..") def out_to_rgb(out,PALETTE,CLASSES): palette = np.array(PALETTE) assert palette.shape[0] == len(CLASSES) assert palette.shape[1] == 3 assert len(palette.shape) == 2 color_seg = np.zeros((out.shape[0], out.shape[1], 3), dtype=np.uint8) for label, color in enumerate(palette): color_seg[out == label, :] = color tran=transforms.ToTensor() color_seg=tran(color_seg) return color_seg def out_to_rgb_np(out,PALETTE,CLASSES): palette = np.array(PALETTE) assert palette.shape[0] == len(CLASSES) assert palette.shape[1] == 3 assert len(palette.shape) == 2 color_seg = np.zeros((out.shape[0], out.shape[1], 3), dtype=np.uint8) for label, color in enumerate(palette): color_seg[out == label, :] = color return color_seg
时间: 2024-03-04 22:52:10 浏览: 17
这段代码看起来是一个图像分割的函数,输入是一个经过模型预测得到的标签图像 out,以及 PALETTE 和 CLASSES 两个参数,PALETTE 是一个颜色调色板,每个类别都对应一种颜色;CLASSES 是一个类别列表,每个元素对应一个类别。输出是一个 RGB 图像,其中每个像素的颜色根据其对应的类别在 PALETTE 中取得。
其中 out_to_rgb_np 函数是不使用 torchvision.transforms.ToTensor 转换的版本,返回的是 numpy 数组类型的 RGB 图像。而 out_to_rgb 函数则是使用了 ToTensor 转换,返回的是 torch.Tensor 类型的 RGB 图像。
相关问题
import numpy as np import matplotlib.pyplot as plt from mpl_toolkits.mplot3d
import numpy是一个用于科学计算的Python库,它提供了大量用于数组操作和数学计算的函数和工具。使用import numpy as np将numpy库导入为np别名,以便在代码中更方便地使用numpy的函数和工具。
而import matplotlib.pyplot as plt是导入matplotlib库的一种常用方式,matplotlib是一个用于绘制图表和数据可视化的库,import语句将matplotlib.pyplot模块导入为plt别名,以便在代码中更方便地使用matplotlib库的绘图函数和工具。
最后,from mpl_toolkits.mplot3d导入模块同样是用于matplotlib库的一种常见操作,它用于导入mpl_toolkits.mplot3d模块,这是一个用于绘制三维图表和可视化三维数据的工具模块。
综合起来,这些import语句在Python代码中的作用是将numpy和matplotlib库以及其相关的模块导入到代码中,以便使用它们提供的各种函数和工具进行数组操作、数学计算和绘制图表等操作。这样可以极大地方便数据科学家、工程师和研究人员们对数据的处理和可视化工作。
import numpy as np from IPython import embed from openvino.inference_engine import IECore
这是一段 Python 代码,其中包含了一些 Python 语言的模块和库的导入操作。具体含义如下:
- `import numpy as np`:导入 Python 的 numpy 模块,并将其重命名为 np,用于进行数值计算和矩阵运算。
- `from IPython import embed`:从 IPython 模块中导入 embed 方法,用于在代码中插入交互式的 Python 解释器,方便调试和测试。
- `from openvino.inference_engine import IECore`:从 openvino.inference_engine 模块中导入 IECore 类,用于在 Intel OpenVINO Toolkit 中进行推理和部署。
这段代码中的导入操作并没有实际的功能,只是为了在后续的代码中使用这些模块和库的功能。
相关推荐
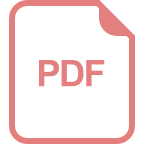
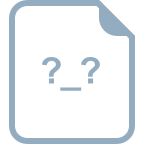
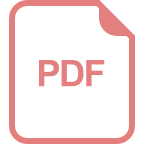












