python从excel中可视化画矩形波的标签及注释及线的颜色和宽度设置
时间: 2024-02-22 19:56:08 浏览: 17
要在Python中从Excel中可视化绘制矩形波形并设置标签和注释,您可以使用以下步骤:
1.使用pandas库读取Excel文件,将数据转换为DataFrame格式。
```python
import pandas as pd
df = pd.read_excel('filename.xlsx')
```
2.使用matplotlib库绘制矩形波形。
```python
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
for i, row in df.iterrows():
ax.fill_between([row['start'], row['end']], [0, 0], [1, 1], color=row['color'], lw=row['line_width'], alpha=0.5)
```
3.设置标签和注释。
```python
for i, row in df.iterrows():
ax.annotate(row['label'], xy=((row['start']+row['end'])/2, 0.5), ha='center', va='center')
```
在这个例子中,我们假设Excel文件包含以下列:start(矩形波开始的位置),end(矩形波结束的位置),color(线的颜色),line_width(线的宽度)和label(矩形波的标签)。
请注意,您需要根据您的数据格式和要求进行适当的调整。
相关问题
python从excel中可视化画矩形波的标签及注释怎么设置
你可以使用Python中的pandas和matplotlib库来从Excel中读取数据,并将其可视化为矩形波形。以下是一个简单的示例代码:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 读取Excel文件
data = pd.read_excel('data.xlsx')
# 将数据转换为矩形波形
fig, ax = plt.subplots()
ax.broken_barh([(start, end-start) for start, end in zip(data['Start'], data['End'])], (0, 1), facecolors='blue')
# 设置标签和注释
plt.title('Rectangular Waveform')
plt.xlabel('Time')
plt.ylabel('Value')
for i, (start, end, label) in enumerate(zip(data['Start'], data['End'], data['Label'])):
ax.annotate(label, xy=((start+end)/2, 0.5), ha='center', va='center')
ax.annotate(f'{end-start}ms', xy=(start, 0.2), ha='left', va='center')
# 显示图形
plt.show()
```
在此代码中,我们首先使用pandas库读取Excel文件中的数据。然后,我们使用matplotlib库的`broken_barh()`函数将数据转换为矩形波形,并使用`facecolors`参数设置矩形波形的颜色。接下来,我们使用`annotate()`函数添加标签和注释。最后,我们使用`show()`函数显示图形。
python中可视化fig, ax = plt.subplots()画矩形波怎么设置横坐标之间的间距宽度及显示出每个横坐标,怎么设置加文本标签和注释,
要设置矩形波的横坐标之间的间距宽度,可以使用 `ax.bar()` 函数,其中 `width` 参数控制矩形的宽度, `align` 参数控制矩形的对齐方式。要显示每个横坐标,可以使用 `ax.set_xticks()` 函数设置横坐标的刻度位置, `ax.set_xticklabels()` 函数设置刻度标签。要加文本标签和注释,可以使用 `ax.text()` 函数和 `ax.annotate()` 函数。以下是示例代码:
```python
import matplotlib.pyplot as plt
# 生成矩形波数据
x = [1, 2, 3, 4, 5]
y = [0, 1, 0, 1, 0]
# 创建画布和坐标轴
fig, ax = plt.subplots()
# 绘制矩形波
ax.bar(x, y, width=0.6, align='center')
# 设置横坐标刻度和标签
ax.set_xticks(x)
ax.set_xticklabels(['A', 'B', 'C', 'D', 'E'])
# 添加文本标签和注释
ax.text(1.5, 0.5, 'On', fontsize=14, ha='center', va='center')
ax.text(3.5, 0.5, 'On', fontsize=14, ha='center', va='center')
ax.annotate('Off', xy=(2.5, 0), xytext=(2.5, -0.5),
fontsize=14, ha='center', va='center',
arrowprops=dict(arrowstyle='->', connectionstyle='arc3'))
# 显示图形
plt.show()
```
其中 `ax.text()` 函数用于添加文本标签,第一个参数是文本的横坐标,第二个参数是文本的纵坐标,第三个参数是文本内容。 `ax.annotate()` 函数用于添加注释,第一个参数是注释的文本内容,`xy` 参数是注释箭头指向的位置, `xytext` 参数是注释文本的位置。 `arrowprops` 参数用于设置注释箭头的样式和连接方式。
相关推荐
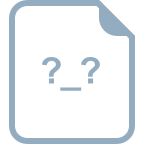
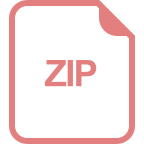






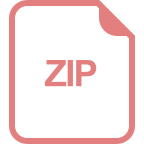
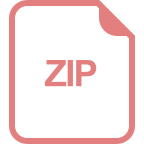
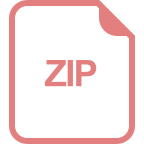
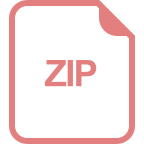
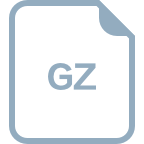
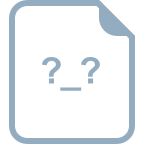