python 有理数类
时间: 2023-09-28 16:10:20 浏览: 139
是的,Python 有一个 fractions 模块,其中包含一个 Fraction 类来处理有理数。Fraction 类可以表示分数,可以进行加减乘除等常见的数学运算,并且可以自动化简分数。以下是一个简单的示例:
```python
from fractions import Fraction
x = Fraction(3, 4) # 创建分数 3/4
y = Fraction(1, 2) # 创建分数 1/2
print(x + y) # 输出 5/4
print(x - y) # 输出 1/4
print(x * y) # 输出 3/8
print(x / y) # 输出 3/2
```
除了 Fraction 类之外,Python 还有一个 decimal 模块,其中包含 Decimal 类,可以处理十进制数值,包括小数和无限精度的有理数。
相关问题
python实现有理数类
Python可以通过定义一个有理数类来实现有理数的操作。下面是一个简单的Python有理数类的示例:
```python
class Rational:
def __init__(self, numerator, denominator):
self.numerator = numerator
self.denominator = denominator
def __str__(self):
return f"{self.numerator}/{self.denominator}"
def __add__(self, other):
if isinstance(other, Rational):
new_numerator = self.numerator * other.denominator + other.numerator * self.denominator
new_denominator = self.denominator * other.denominator
return Rational(new_numerator, new_denominator)
else:
raise TypeError("Unsupported operand type: +")
def __sub__(self, other):
if isinstance(other, Rational):
new_numerator = self.numerator * other.denominator - other.numerator * self.denominator
new_denominator = self.denominator * other.denominator
return Rational(new_numerator, new_denominator)
else:
raise TypeError("Unsupported operand type: -")
def __mul__(self, other):
if isinstance(other, Rational):
new_numerator = self.numerator * other.numerator
new_denominator = self.denominator * other.denominator
return Rational(new_numerator, new_denominator)
else:
raise TypeError("Unsupported operand type: *")
def __truediv__(self, other):
if isinstance(other, Rational):
new_numerator = self.numerator * other.denominator
new_denominator = self.denominator * other.numerator
return Rational(new_numerator, new_denominator)
else:
raise TypeError("Unsupported operand type: /")
```
上述代码定义了一个有理数类`Rational`,它包含了有理数的分子和分母属性,并重载了加法、减法、乘法和除法运算符。通过创建`Rational`类的实例,可以进行有理数的运算。
定义有理数类Python
在Python中,我们可以自定义一个有理数类(Rational Number Class),通过继承内置的`numbers`模块中的` Rational`类或直接创建一个类来实现。下面是一个简单的例子,展示如何定义一个名为`CustomRational`的有理数类:
```python
from fractions import Fraction
class CustomRational:
def __init__(self, numerator, denominator):
# 检查分母是否为0,避免除以零错误
if denominator == 0:
raise ValueError("Denominator cannot be zero.")
self.numerator = numerator
self.denominator = abs(denominator) # 取绝对值以确保正数表示
def __add__(self, other):
return CustomRational(self.numerator * other.denominator + other.numerator * self.denominator,
self.denominator * other.denominator)
def __sub__(self, other):
return CustomRational(self.numerator * other.denominator - other.numerator * self.denominator,
self.denominator * other.denominator)
def __mul__(self, other):
return CustomRational(self.numerator * other.numerator, self.denominator * other.denominator)
def __truediv__(self, other):
if isinstance(other, CustomRational):
return CustomRational(self.numerator * other.denominator, self.denominator * other.numerator)
else:
return CustomRational(self.numerator * other, self.denominator)
def __str__(self):
return f"{self.numerator}/{self.denominator}"
# 示例用法
r1 = CustomRational(4, 7)
r2 = CustomRational(3, 5)
print(r1 + r2) # 输出:(29/35)
```
在这个`CustomRational`类中,我们实现了加法 (`__add__`)、减法 (`__sub__`)、乘法 (`__mul__`) 和除法 (`__truediv__`) 这些基本操作,以及字符串转换 (`__str__`) 方法。用户可以创建这个类的实例,并像处理标准的有理数一样使用它们。
阅读全文
相关推荐
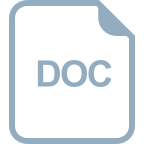
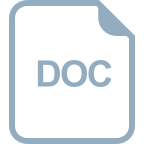
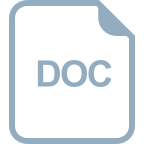
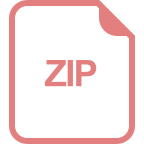







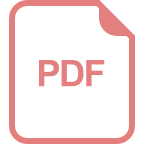
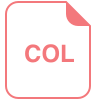



