帮我写一个是用word2vec提取特征,并使用PCA降维的文本分类任务的代码
时间: 2023-08-28 18:25:51 浏览: 152
好的,这里是一个使用word2vec提取特征并使用PCA降维的文本分类任务的Python代码示例:
```python
import numpy as np
import pandas as pd
import gensim
from sklearn.model_selection import train_test_split
from sklearn.decomposition import PCA
from keras.models import Sequential
from keras.layers import Dense, Dropout
from keras.layers import LSTM, Embedding
from keras.preprocessing.text import Tokenizer
from keras.preprocessing.sequence import pad_sequences
from keras.utils import to_categorical
# Load the dataset
data = pd.read_csv('dataset.csv')
# Split the dataset into training and testing sets
train_data, test_data = train_test_split(data, test_size=0.2, random_state=42)
# Load the pre-trained Word2Vec model
model = gensim.models.KeyedVectors.load_word2vec_format('word2vec.bin', binary=True)
# Tokenize the text
tokenizer = Tokenizer()
tokenizer.fit_on_texts(data['text'])
# Convert the text into sequences of word indices
train_sequences = tokenizer.texts_to_sequences(train_data['text'])
test_sequences = tokenizer.texts_to_sequences(test_data['text'])
# Pad the sequences to a fixed length
max_seq_length = 100
train_sequences = pad_sequences(train_sequences, maxlen=max_seq_length)
test_sequences = pad_sequences(test_sequences, maxlen=max_seq_length)
# Create the embedding matrix
embedding_dim = 300
vocab_size = len(tokenizer.word_index) + 1
embedding_matrix = np.zeros((vocab_size, embedding_dim))
for word, i in tokenizer.word_index.items():
if word in model:
embedding_matrix[i] = model[word]
# Convert the labels into one-hot encoded vectors
train_labels = to_categorical(train_data['label'])
test_labels = to_categorical(test_data['label'])
# Extract the features using the pre-trained Word2Vec model
train_features = np.zeros((len(train_sequences), embedding_dim))
for i, sequence in enumerate(train_sequences):
for j, word_index in enumerate(sequence):
if word_index != 0:
train_features[i][j] = np.mean(model[tokenizer.index_word[word_index]])
test_features = np.zeros((len(test_sequences), embedding_dim))
for i, sequence in enumerate(test_sequences):
for j, word_index in enumerate(sequence):
if word_index != 0:
test_features[i][j] = np.mean(model[tokenizer.index_word[word_index]])
# Perform PCA on the features
pca = PCA(n_components=100)
train_features = pca.fit_transform(train_features)
test_features = pca.transform(test_features)
# Define the model architecture
model = Sequential()
model.add(Dense(128, activation='relu', input_dim=100))
model.add(Dropout(0.5))
model.add(Dense(train_labels.shape[1], activation='softmax'))
# Compile the model
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
# Train the model
model.fit(train_features, train_labels, batch_size=128, epochs=10, validation_data=(test_features, test_labels))
```
这段代码与先前的示例非常相似,但是在提取特征后使用PCA进行降维。在这个示例中,我们使用一个简单的2层神经网络来对文本进行分类。
阅读全文
相关推荐
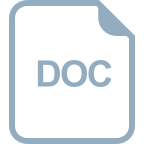
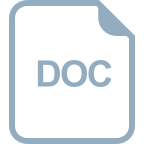
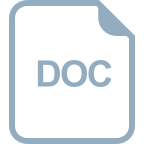

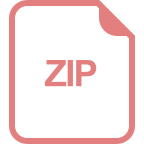
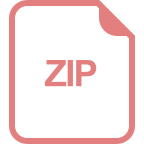
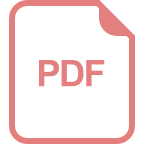
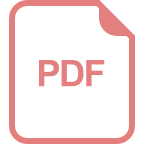
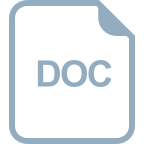
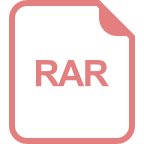
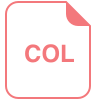
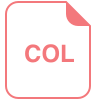
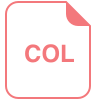
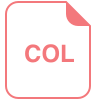
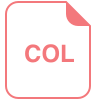
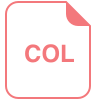
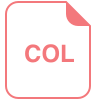

