std::string判断是否为空
时间: 2023-11-14 08:07:16 浏览: 61
可以使用`empty()`函数来判断一个`std::string`是否为空,如果为空,则返回`true`,否则返回`false`。示例代码如下:
```c++
#include <iostream>
#include <string>
int main()
{
std::string str1 = "";
std::string str2 = "Hello, world!";
if (str1.empty())
{
std::cout << "str1 is empty." << std::endl;
}
else
{
std::cout << "str1 is not empty." << std::endl;
}
if (str2.empty())
{
std::cout << "str2 is empty." << std::endl;
}
else
{
std::cout << "str2 is not empty." << std::endl;
}
return 0;
}
```
输出结果为:
```
str1 is empty.
str2 is not empty.
```
相关问题
std::string 如何判断是否为空
std::string可以通过以下方法来判断是否为空:
1. 使用empty()函数。如果该字符串为空,则empty()函数将返回true。例如:
```c++
std::string str1 = "";
if (str1.empty()) {
std::cout << "str1 is empty." << std::endl;
}
```
2. 使用length()函数。如果该字符串长度为0,则说明该字符串为空。例如:
```c++
std::string str2 = "";
if (str2.length() == 0) {
std::cout << "str2 is empty." << std::endl;
}
```
3. 与空串""比较,如果相等则为空。例如:
```c++
std::string str3 = "";
if (str3 == "") {
std::cout << "str3 is empty." << std::endl;
}
```
以上三种方法中,empty()函数是效率最高也是最常用的一种方法。但不能使用 str==NULL 来判断,NULL一般只拿和指针做比较或者赋给指针,std::string是类,传参进函数时调用默认构造函数已经初始化了,并且已经是对象了,它不可能为NULL,也不能和NULL比较。不能使用 str.c_str()==" " 来判断,这种方式会将字符串转换为C-style字符串,然后和空串进行比较,但是由于在C++中字符串可以包含空字符,因此这种方式并不可靠。
std::string如何判断是否为空字符串
可以使用`empty()`函数判断`std::string`是否为空字符串,该函数返回一个`bool`类型的值,如果字符串为空,则返回`true`,否则返回`false`。例如:
```c++
std::string str = "";
if(str.empty()){
std::cout << "字符串为空" << std::endl;
} else {
std::cout << "字符串不为空" << std::endl;
}
```
输出结果为:`字符串为空`
阅读全文
相关推荐













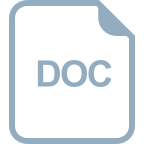
