C# 如何通讯使用 IEC61850 ?
时间: 2023-07-20 17:30:25 浏览: 410
IEC 61850 是一种用于智能电网和电力系统自动化的通信协议标准。在 C# 中,可以使用 MMS 或 GOOSE 协议与 IEC 61850 进行通信。以下是通信步骤:
1. 创建一个 MMS 或 GOOSE 协议的客户端对象。
2. 使用客户端对象连接到 IEC 61850 服务器。
3. 使用客户端对象发送和接收 MMS 或 GOOSE 报文。
4. 处理接收的数据并进行相应的操作。
以下是一个使用 MMS 协议通信的示例代码:
```csharp
using System;
using System.Net.Sockets;
using System.Text;
namespace IEC61850Communication
{
class MMSClient
{
private TcpClient client;
private NetworkStream stream;
public void Connect(string ip, int port)
{
client = new TcpClient(ip, port);
stream = client.GetStream();
}
public void SendMMS(byte[] data)
{
stream.Write(data, 0, data.Length);
}
public byte[] ReceiveMMS()
{
byte[] buffer = new byte[1024];
int bytesRead = stream.Read(buffer, 0, buffer.Length);
byte[] data = new byte[bytesRead];
Array.Copy(buffer, data, bytesRead);
return data;
}
}
}
```
使用 MMS 协议时,需要构建 MMS 报文。可以使用第三方库或手动构建报文。以下是一个手动构建 MMS 报文的示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace IEC61850Communication
{
class MMSMessage
{
private byte[] bytes;
public MMSMessage(byte[] bytes)
{
this.bytes = bytes;
}
public byte[] GetBytes()
{
return bytes;
}
public static byte[] BuildReadRequest(string variableName)
{
List<byte> message = new List<byte>();
message.AddRange(new byte[] { 0x60, 0x0a, 0x01, 0x01 }); // Message header
message.AddRange(new byte[] { 0xa0, 0x03, 0x02, 0x01, 0x00 }); // Invoke ID
message.AddRange(new byte[] { 0x80, 0x02, 0x07, 0x00 }); // Service
message.AddRange(new byte[] { 0x6f, 0x0c }); // Variable specification
message.AddRange(new byte[] { 0x60, 0x0a, 0x80, 0x00 }); // Variable access specification
byte[] variableNameBytes = Encoding.ASCII.GetBytes(variableName);
message.Add((byte)variableNameBytes.Length); // Variable name length
message.AddRange(variableNameBytes); // Variable name
return message.ToArray();
}
public static byte[] BuildWriteRequest(string variableName, byte[] value)
{
List<byte> message = new List<byte>();
message.AddRange(new byte[] { 0x60, 0x0a, 0x01, 0x01 }); // Message header
message.AddRange(new byte[] { 0xa0, 0x03, 0x02, 0x01, 0x00 }); // Invoke ID
message.AddRange(new byte[] { 0x80, 0x02, 0x06, 0x00 }); // Service
message.AddRange(new byte[] { 0x6f, 0x0c }); // Variable specification
message.AddRange(new byte[] { 0x60, 0x0a, 0xc0, 0x00 }); // Variable access specification
byte[] variableNameBytes = Encoding.ASCII.GetBytes(variableName);
message.Add((byte)variableNameBytes.Length); // Variable name length
message.AddRange(variableNameBytes); // Variable name
message.AddRange(new byte[] { 0x87, 0x01, (byte)value.Length }); // Data
message.AddRange(value); // Data
return message.ToArray();
}
}
}
```
使用 MMS 协议时,需要了解 MMS 报文格式和协议的具体细节。
阅读全文
相关推荐
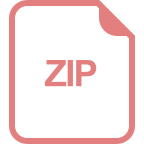
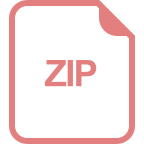
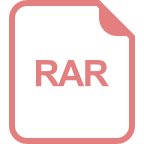
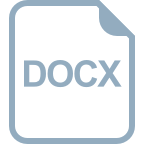
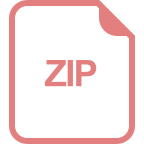
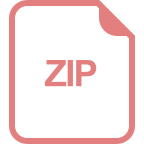
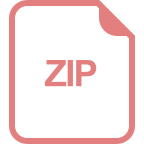
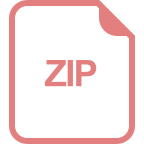
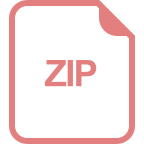
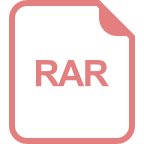
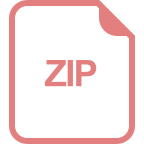
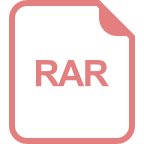
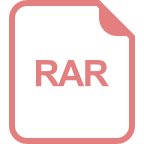
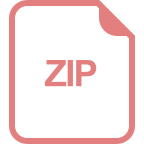
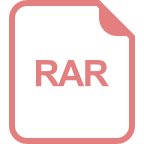
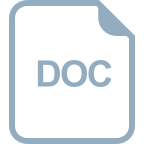
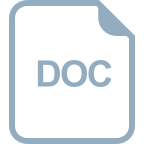