java function retry wrapper
时间: 2023-10-25 14:09:49 浏览: 164
可以为您提供一个简单的 Java 函数重试包装器的实现,如下所示:
```java
public class RetryWrapper {
public static <T> T retry(int maxAttempts, Callable<T> callable) throws Exception {
int attempt = 0;
while (true) {
try {
return callable.call();
} catch (Exception e) {
if (++attempt >= maxAttempts) {
throw e;
}
}
}
}
}
```
调用示例:
```java
int result = RetryWrapper.retry(3, () -> {
// your code here
return someValue;
});
```
您可以将要重试的代码放在 lambda 表达式中,然后调用 `RetryWrapper.retry` 函数即可。函数将尝试执行代码,如果发生异常则会重新尝试执行,最多尝试 `maxAttempts` 次,如果所有尝试均失败,则将抛出异常。
相关问题
Implement retry in Java for http request
Sure, here's an example of how to implement retry in Java for an HTTP request using the Apache HttpClient library:
```java
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.protocol.HttpContext;
import java.io.IOException;
public class HttpRequestRetry {
private static final int MAX_RETRIES = 3;
public static void main(String[] args) throws IOException {
HttpClient httpClient = HttpClientBuilder.create().build();
HttpGet httpGet = new HttpGet("https://www.example.com");
int retryCount = 0;
boolean success = false;
while (retryCount < MAX_RETRIES && !success) {
try {
httpClient.execute(httpGet, (HttpContext) null);
success = true;
} catch (IOException e) {
System.out.println("Request failed, retrying...");
retryCount++;
}
}
if (success) {
System.out.println("Request succeeded after " + retryCount + " retries.");
} else {
System.out.println("Request failed after " + retryCount + " retries.");
}
}
}
```
In this example, we create an `HttpClient` instance using the `HttpClientBuilder` class, and then create an `HttpGet` request object for the URL we want to retrieve. We then loop through the request up to a maximum number of retries (in this case, 3), attempting the request each time and catching any `IOException` that is thrown. If the request succeeds, we set the `success` flag to true and exit the loop. If the request fails after the maximum number of retries, we print a failure message.
java中一个方法十个retry如何优化
在Java中,如果你需要在一个方法中处理可能会抛出异常的情况,并且为了达到某种目标多次尝试(比如`retry`),通常会采用循环加上异常处理的方式来实现。这里是一个简单的示例:
```java
public class RetryMethod {
private int maxRetries = 5; // 最大重试次数
public void doSomething() throws Exception {
for (int i = 0; i < maxRetries; i++) {
try {
// 尝试执行方法
executeTask();
if (isSucceeded()) { // 如果任务成功,则退出循环
return;
}
} catch (Exception e) {
// 捕获异常并记录日志
logRetryException(e);
// 根据情况调整延迟时间再尝试
Thread.sleep(BackoffStrategy.calculateDelay(i)); // 使用回退策略调整延时
}
}
// 当所有重试都失败后,抛出最终异常
throw new MaxRetriesExceededException("Max retries exceeded after " + maxRetries + " attempts.");
}
private void executeTask() {
// 你的实际业务操作...
}
private boolean isSucceeded() {
// 判断任务是否成功的条件...
}
private void logRetryException(Exception e) {
// 日志记录异常信息...
}
}
```
在这个例子中,我们使用了一个简单的回退策略,即每次失败后增加一些固定的延迟,直到达到最大重试次数。如果所有重试都失败了,就抛出一个自定义的`MaxRetriesExceededException`。
**
阅读全文
相关推荐
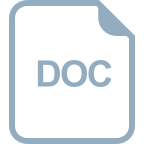
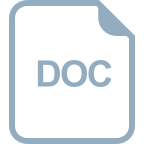
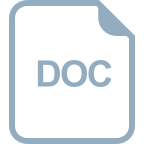
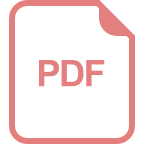
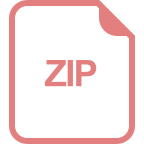
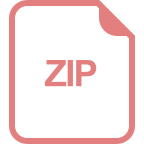
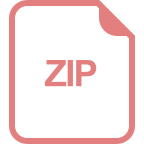
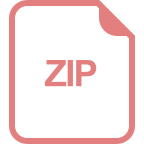








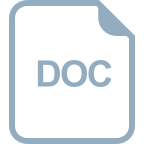