利用python3.8语言实现堆栈自编码器算法在MNISTS手写数字识别,并且 选取一定数目的样本分别作为训练和测试数据,固定一组参数设置,实现对MNIST手写数字有效识别,并基于网络训练过程中的可视化图分析识别性能随迭代次数变化情况, 尝试修改训练/测试样本(数目)、隐藏层神经元节点数目、隐藏层数目、学习率等参数,获得不同场景下的识别精度
时间: 2024-04-20 22:23:41 浏览: 95
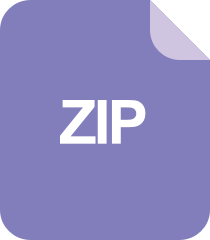
国三作品-基于深度学习平台利用识别数字算法进行样本数据训练和测试python代码.zip
您可以使用Python 3.8和相关的库(如TensorFlow、Keras)来实现堆栈自编码器算法在MNIST手写数字识别上的任务。以下是一种可能的实现方法:
1. 导入所需的库:
```python
import numpy as np
import matplotlib.pyplot as plt
from tensorflow import keras
from tensorflow.keras.datasets import mnist
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, Dense
```
2. 加载MNIST数据集,并进行预处理:
```python
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
# 数据归一化
train_images = train_images.astype('float32') / 255.
test_images = test_images.astype('float32') / 255.
# 数据展平
train_images = train_images.reshape((len(train_images), np.prod(train_images.shape[1:])))
test_images = test_images.reshape((len(test_images), np.prod(test_images.shape[1:])))
```
3. 定义堆栈自编码器模型:
```python
input_dim = train_images.shape[1]
# 编码器
input_img = Input(shape=(input_dim,))
encoded = Dense(128, activation='relu')(input_img)
encoded = Dense(64, activation='relu')(encoded)
encoded = Dense(32, activation='relu')(encoded)
# 解码器
decoded = Dense(64, activation='relu')(encoded)
decoded = Dense(128, activation='relu')(decoded)
decoded = Dense(input_dim, activation='sigmoid')(decoded)
# 构建自编码器模型
autoencoder = Model(input_img, decoded)
autoencoder.compile(optimizer='adam', loss='binary_crossentropy')
```
4. 进行模型训练:
```python
epochs = 20
batch_size = 256
history = autoencoder.fit(train_images, train_images,
epochs=epochs,
batch_size=batch_size,
shuffle=True,
validation_data=(test_images, test_images))
```
5. 分析训练过程中的可视化图:
```python
# 绘制训练集和测试集的损失曲线
plt.plot(history.history['loss'])
plt.plot(history.history['val_loss'])
plt.title('Model Loss')
plt.ylabel('Loss')
plt.xlabel('Epoch')
plt.legend(['Train', 'Test'], loc='upper right')
plt.show()
```
6. 使用训练好的模型进行预测和评估性能:
```python
# 对测试集进行预测
decoded_imgs = autoencoder.predict(test_images)
# 随机选择几个样本进行可视化比较
n = 10
plt.figure(figsize=(20, 4))
for i in range(n):
# 原始图像
ax = plt.subplot(2, n, i + 1)
plt.imshow(test_images[i].reshape(28, 28))
plt.gray()
ax.get_xaxis().set_visible(False)
ax.get_yaxis().set_visible(False)
# 重构图像
ax = plt.subplot(2, n, i + 1 + n)
plt.imshow(decoded_imgs[i].reshape(28, 28))
plt.gray()
ax.get_xaxis().set_visible(False)
ax.get_yaxis().set_visible(False)
plt.show()
# 计算重构图像与原始图像之间的均方根误差
mse = np.mean(np.power(test_images - decoded_imgs, 2), axis=1)
```
通过修改训练/测试样本数目、隐藏层神经元节点数目、隐藏层数目、学习率等参数,您可以获得不同场景下的识别精度。可以通过调整这些参数并观察性能的变化来找到最佳的参数设置。请注意,这只是一种实现方式,您可以根据需要进行进一步的调整和改进。
阅读全文
相关推荐
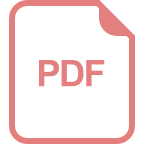
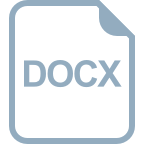















