如果字体文件放在res 的font文件夹下应该怎么使用Typeface方法
时间: 2024-02-03 17:15:44 浏览: 18
如果您的字体文件放在 `res/font` 文件夹下,您可以通过以下代码来使用 Typeface 方法:
```java
// 获取字体文件的路径
Typeface typeface = Typeface.createFromAsset(getAssets(), "font/your_font_file.ttf");
// 将字体应用到 TextView 上
TextView textView = findViewById(R.id.your_text_view);
textView.setTypeface(typeface);
```
其中 `"font/your_font_file.ttf"` 中的 `"font/"` 是必须的,因为它指定了字体文件的路径。如果您将字体文件放在其他文件夹下,可以相应地修改该字符串。
相关问题
字体不放在assets目录下是否可以设置字体
在Android中,字体可以通过将其放置在assets目录下或者使用系统自带的字体进行设置。如果你想使用非系统自带的字体,那么需要将字体文件放置在assets目录下,然后使用Typeface类进行设置。具体实现步骤如下:
1. 将字体文件放置在assets目录下,例如放在assets/fonts目录下。
2. 在代码中使用Typeface类加载字体文件,例如:
```
Typeface typeface = Typeface.createFromAsset(getAssets(), "fonts/xxx.ttf");
```
3. 将Typeface对象设置给TextView或其他控件,例如:
```
textView.setTypeface(typeface);
```
这样就可以设置非系统自带的字体了。注意,在使用Typeface类加载字体文件时,需要使用正确的文件名和文件路径。
Android自定义字体文件
要在Android应用程序中使用自定义字体文件,请按照以下步骤操作:
1. 将字体文件放置在您的项目的“assets”文件夹中。如果该文件夹不存在,请创建它。
2. 在您的活动或应用程序类中创建一个“Typeface”对象,以便您可以使用该字体。
```java
Typeface customFont = Typeface.createFromAsset(getAssets(), "yourfont.ttf");
```
3. 在您的布局文件或代码中应用此字体。
- 布局xml文件:
```xml
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
android:textSize="24sp"
android:typeface="yourcustomfont.ttf" />
```
- 代码:
```java
TextView textView = (TextView) findViewById(R.id.textView1);
textView.setTypeface(customFont);
```
现在,您的Android应用程序中的文本将使用您的自定义字体文件。
相关推荐
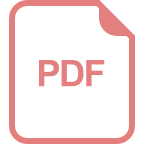
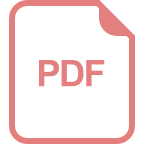
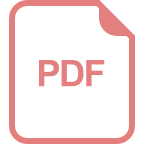












