Java 中怎么实现 cas
时间: 2023-06-18 11:07:35 浏览: 50
CAS (Compare And Swap) 是一种常见的并发控制方式,它用于保证多个线程同时访问共享资源时,只有一个线程能够修改共享资源并且其他线程能够得到最新的修改后的值。在 Java 中,CAS 操作可以通过使用 Atomic 类来实现。通过使用 Atomic 类提供的 compareAndSet() 方法,实现 CAS 操作,其中 compareAndSet() 方法是原子性的,可以保证只有一个线程能够成功修改共享变量的值。例如:
```
AtomicInteger count = new AtomicInteger(0);
count.compareAndSet(0,1); // 只有 count 的值是 0 的时候才会将其更新为 1
```
当 count 的值为 0 时,compareAndSet() 方法会将 count 的值更新为 1;当 count 的值不为 0 时,compareAndSet() 方法不会更新 count 的值。这样可以保证 count 的值始终是最新的,避免多线程并发访问时产生冲突。
相关问题
java线程中cas
CAS(Compare and Swap)是一种多线程同步的机制,用于实现无锁的并发操作。
在Java线程中,CAS通常通过java.util.concurrent.atomic包中的原子类来实现。这些原子类提供了一组原子操作,比如AtomicInteger、AtomicLong和AtomicReference等。
CAS操作包含三个操作数:内存位置(通常是一个变量的内存地址)、预期值和新值。它的执行过程如下:
1. 读取内存位置的当前值作为预期值。
2. 比较预期值与内存位置的当前值,如果相等,则将新值写入内存位置;如果不相等,则说明其他线程已经修改了内存位置的值,需要重新读取当前值并重试。
3. 返回操作是否成功。
CAS操作是原子的,可以保证在多线程环境下对共享变量的原子性操作。它避免了使用传统的锁机制所带来的性能损失。
在Java线程中使用CAS操作可以实现一些常见的并发模型,比如无锁算法、自旋锁和非阻塞数据结构等。但需要注意的是,CAS操作并不适用于所有情况,它对于比较简单的操作比较高效,但对于复杂的操作可能存在ABA问题(即一个值被修改两次后又变回原来的值)和性能问题。
因此,在使用CAS操作时需要仔细考虑具体的应用场景,权衡其优势和限制。
java CAS代码实现
CAS(Compare And Swap)是一种并发控制的机制,可以保证多个线程同时对同一个变量进行操作时,只有一个线程能够成功修改变量的值。以下是一个简单的 Java CAS 代码实现:
```java
public class CASDemo {
private volatile int value; // 需要进行原子操作的变量
public synchronized int getValue() {
return value;
}
public synchronized boolean compareAndSet(int expectedValue, int newValue) {
if (value == expectedValue) {
value = newValue;
return true;
}
return false;
}
}
```
在上面的代码中,`value` 是需要进行原子操作的变量,使用 `volatile` 关键字修饰,保证了多线程之间的可见性。`getValue()` 方法用于获取当前变量的值,使用 `synchronized` 关键字修饰,保证了多线程之间的互斥性。`compareAndSet()` 方法用于比较当前变量的值是否等于期望值,如果相等则修改变量的值为新值,并返回 `true`,否则返回 `false`。在 `compareAndSet()` 方法中也使用了 `synchronized` 关键字,保证了多线程之间的互斥性。
相关推荐












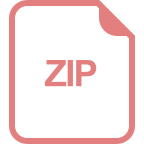