给出C函数,完成读取iptable中所有的input规则,逐条获取-p、-s、--sport、--sport、-j参数,存储到二维数组中,给出c函数
时间: 2024-03-27 21:41:17 浏览: 85
以下是一个简单的C函数,可以读取iptables中所有的input规则,逐条获取-p、-s、--sport、--sport、-j参数,并将它们存储到二维数组中:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_RULES 100
#define MAX_PARAMS 6
#define MAX_PARAM_LENGTH 20
char iptables[MAX_RULES][MAX_PARAMS][MAX_PARAM_LENGTH];
int read_iptables() {
FILE *fp;
char rule[100];
int rule_count = 0;
fp = popen("iptables -L INPUT --line-numbers -n", "r");
if (fp == NULL) {
printf("Failed to execute command\n");
return -1;
}
while (fgets(rule, sizeof(rule), fp) != NULL) {
if (strncmp(rule, "Chain", 5) == 0) {
continue;
}
if (strncmp(rule, "num", 3) == 0) {
continue;
}
char *p = strtok(rule, " ");
int param_count = 0;
while (p != NULL) {
if (strncmp(p, "-p", 2) == 0 ||
strncmp(p, "-s", 2) == 0 ||
strncmp(p, "--sport", 7) == 0 ||
strncmp(p, "--dport", 7) == 0 ||
strncmp(p, "-j", 2) == 0) {
strncpy(iptables[rule_count][param_count], p, MAX_PARAM_LENGTH);
param_count++;
}
p = strtok(NULL, " ");
}
rule_count++;
}
pclose(fp);
return rule_count;
}
int main() {
int rule_count = read_iptables();
for (int i = 0; i < rule_count; i++) {
for (int j = 0; j < MAX_PARAMS; j++) {
printf("%s ", iptables[i][j]);
}
printf("\n");
}
return 0;
}
```
这个函数使用了popen函数来执行命令,然后使用fgets函数逐行读取iptables的输出。对于每一行,函数使用strtok函数将其分割成单独的参数,然后检查每个参数是否是我们需要的参数(-p、-s、--sport、--dport、-j),如果是,就将其存储到二维数组中。最后,函数返回规则数量,方便后面的处理。
阅读全文
相关推荐
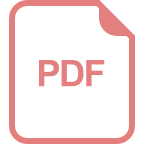
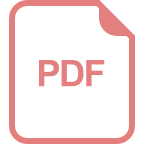
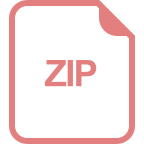








