vector.assign
时间: 2023-10-23 16:13:05 浏览: 61
The `vector.assign` function is used to assign new values to a vector. It replaces the existing elements of the vector with the new set of elements provided as arguments.
Syntax:
```
vector.assign(count, value);
vector.assign(startIterator, endIterator);
vector.assign(initializerList);
```
Parameters:
- `count`: the number of elements to be inserted into the vector.
- `value`: the value to be assigned to each element being inserted into the vector.
- `startIterator`: an iterator pointing to the beginning of a range of elements to be inserted into the vector.
- `endIterator`: an iterator pointing to the end of a range of elements to be inserted into the vector.
- `initializerList`: an initializer list containing the elements to be inserted into the vector.
Return value:
None.
Example:
```
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> vec;
// Assigning 5 elements with value 10
vec.assign(5, 10);
// Displaying the elements of the vector
for (int i = 0; i < vec.size(); i++) {
cout << vec[i] << " ";
}
cout << endl;
// Assigning elements from a range
int arr[] = { 1, 2, 3, 4, 5 };
vec.assign(arr, arr + 5);
// Displaying the elements of the vector
for (int i = 0; i < vec.size(); i++) {
cout << vec[i] << " ";
}
cout << endl;
// Assigning elements using an initializer list
vec.assign({ 6, 7, 8, 9, 10 });
// Displaying the elements of the vector
for (int i = 0; i < vec.size(); i++) {
cout << vec[i] << " ";
}
cout << endl;
return 0;
}
```
Output:
```
10 10 10 10 10
1 2 3 4 5
6 7 8 9 10
```
阅读全文
相关推荐
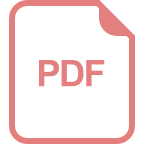
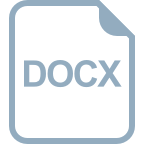
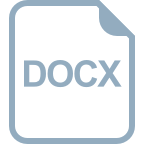










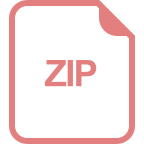
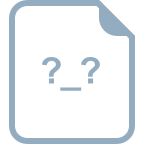