string去除转义 c++
时间: 2023-07-19 14:24:04 浏览: 89
在 C++ 中,我们可以使用反斜杠字符 '\' 来表示特殊字符,这些特殊字符也称为转义字符。如果我们想要在一个字符串中包含反斜杠字符 '\',我们需要使用双反斜杠 '\\' 来表示。
如果我们想要去除字符串中的转义字符,可以使用以下代码:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "hello\\nworld\\t";
string result;
for (int i = 0; i < str.length(); i++) {
if (str[i] == '\\') {
i++; // 跳过转义字符
}
result += str[i];
}
cout << result << endl;
return 0;
}
```
在上面的代码中,我们遍历字符串中的每个字符,如果遇到了反斜杠字符 '\',就跳过它的下一个字符。最后将没有转义字符的字符依次添加到结果字符串中。
相关问题
c++如何去除json中的转义符
在 C++ 中,可以使用第三方库如 rapidjson 来解析和处理 JSON 数据。对于解析后的字符串中含有转义符的情况,可以使用库提供的转义符解码函数进行处理。
以下是一个使用 rapidjson 库解析 JSON 数据并去除转义符的示例代码:
```c++
#include <iostream>
#include "rapidjson/document.h"
#include "rapidjson/stringbuffer.h"
#include "rapidjson/writer.h"
using namespace rapidjson;
int main() {
// 解析 JSON 字符串
const char* json = "{\"name\":\"John\\\"Doe\", \"age\":30}";
Document document;
document.Parse(json);
// 从解析后的字符串中获取 name 字段的值并去除转义符
std::string name = document["name"].GetString();
StringBuffer buffer;
Writer<StringBuffer> writer(buffer);
for (auto c : name) {
if (c == '\\' && !name.empty() && name.back() != '\\') {
continue;
}
writer.Put(c);
}
std::string decoded_name = buffer.GetString();
// 输出结果
std::cout << "原始字符串:" << name << std::endl;
std::cout << "去除转义符后:" << decoded_name << std::endl;
return 0;
}
```
在上述代码中,我们首先使用 rapidjson 库解析了一个包含转义符的 JSON 字符串,并从中获取了 name 字段的值。接着,我们使用 rapidjson 提供的 Writer 类来将解码后的字符串写入到一个 StringBuffer 中,最终得到了去除转义符后的字符串。需要注意的是,转义符 \ 在 C++ 中也是一个特殊字符,因此需要进行特殊处理。
C++删除各种转义字符
要在 C++ 中删除各种转义字符,可以使用字符串的替换操作来去除转义字符。以下是一个示例代码:
```cpp
#include <iostream>
#include <string>
std::string removeEscapeCharacters(const std::string& str) {
std::string unescapedStr = str;
// 替换转义字符
size_t found = unescapedStr.find('\\');
while (found != std::string::npos) {
unescapedStr.replace(found, 2, "");
found = unescapedStr.find('\\', found);
}
return unescapedStr;
}
int main() {
std::string str = "Hello\\tWorld!\\nThis is a\\\"test\\\" string.";
std::cout << "Original string: " << str << std::endl;
std::string unescapedStr = removeEscapeCharacters(str);
std::cout << "Unescaped string: " << unescapedStr << std::endl;
return 0;
}
```
输出结果:
```
Original string: Hello\tWorld!\nThis is a\"test\" string.
Unescaped string: HelloWorld!This is a"test" string.
```
在上面的示例中,我们定义了一个函数 `removeEscapeCharacters`,它接受一个字符串作为输入,并创建一个副本 `unescapedStr` 来存储去除转义字符后的字符串。然后使用 `std::string` 类的 `find` 和 `replace` 方法来查找并替换转义字符。使用 `find` 方法找到第一个转义字符的位置,然后使用 `replace` 方法将该转义字符及其后面的字符替换为空字符串。然后继续查找并替换下一个转义字符,直到没有转义字符为止。最后返回已去除转义字符的字符串。
在 `main` 函数中演示了如何使用该函数来去除转义字符,并输出结果。
阅读全文
相关推荐
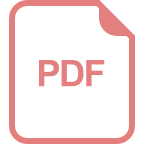
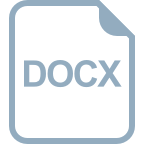
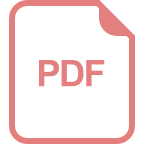
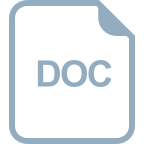
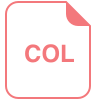
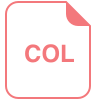
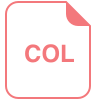
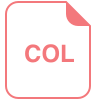
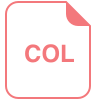
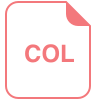




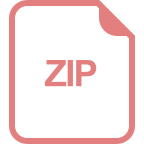