qs = qs.filter(name=request.GET['name']),当前qs查询出来5个QuerySet实例,如何将这5条数据根据name和age去重,并且保证去重后结果不是字典,而是跟qs = qs.filter(name=request.GET['name'])结果类型一致,并且将重复的条数定义为新字段添加在数据中
时间: 2024-02-17 16:59:50 浏览: 33
你可以使用 Django 的 annotate() 和 values() 方法来实现这个需求。具体步骤如下:
1. 首先,通过 values() 方法获取到需要去重的字段,这里是 name 和 age。此时,你会得到一个包含重复数据的 QuerySet。
2. 接着,使用 annotate() 方法对 QuerySet 进行分组和计数操作。具体来说,你可以使用 Count() 和 distinct=True 参数来计算每组数据出现的次数,并且去重。
3. 最后,使用 values() 方法将结果转换为字典列表,然后将计数的结果添加为新字段。
下面是具体代码实现:
```
from django.db.models import Count
qs = qs.filter(name=request.GET['name'])
distinct_qs = qs.values('name', 'age').annotate(count=Count('id', distinct=True)).values('name', 'age', 'count')
```
这段代码会将 qs 中符合条件的数据按照 name 和 age 进行分组,然后计算每组数据的数量,并且去重。最后,将结果转换为字典列表,并且添加了一个新的 count 字段来表示数量。
相关问题
class warehousePeopleBase(ModelAdmin): model = warehousePeople menu_label = '库房人员出入库信息' # ditch this to use verbose_name_plural from model menu_icon = 'site' # change as required list_display = ['name', 'month', 'warehouse', 'subjectMatter'] list_filter = ('name', 'month', 'warehouse',) def apply_prove(self, obj): return format_html("<span>认证完成</span>") apply_prove.short_description = '入库次数' apply_prove.allow_tags = True def get_queryset(self, request): qs = super().get_queryset(request) if 'name' in request.GET and request.GET['name'] != 'none': # 条件查询 qs = qs.filter(name=request.GET['name']) if 'subjectMatter' in self.list_display: self.list_display.remove('subjectMatter') # qs = qs.values('month', 'warehouse').annotate(apply_prove=Count('id')).distinct() # self.list_display = ['name', 'month', 'warehouse', 'apply_prove'] if 'apply_prove' not in self.list_display: self.list_display.append('apply_prove') else: if 'subjectMatter' not in self.list_display: self.list_display.append('subjectMatter') if "apply_prove" in self.list_display: self.list_display.remove('apply_prove') return qs 代码中的qs = qs.filter(name=request.GET['name']),当前qs查询出来5个QuerySet实例,如何根据month和warehouse去重,并且将重复的条数定义为新字段添加在数据中,去重后结果不是字典,而是跟qs = qs.filter(name=request.GET['name'])结果类型一致
可以使用`values`和`annotate`方法来实现按照`month`和`warehouse`字段去重,并且将重复的条数定义为新字段添加在数据中。
可以将以下代码:
```
# qs = qs.filter(name=request.GET['name']).distinct()
```
替换为以下代码:
```
qs = qs.filter(name=request.GET['name']).values('month', 'warehouse').annotate(apply_prove=Count('id')).distinct()
```
这样就可以按照`month`和`warehouse`字段去重,并且将重复的条数定义为新字段`apply_prove`添加在数据中。注意要引入`Count`函数。
def get_queryset(self, request): qs = super().get_queryset(request) result = [] if 'name' in request.GET and request.GET['name'] != 'none': # 条件查询 qs = warehousePeople.objects.filter(name=request.GET['name']) if 'subjectMatter' in self.list_display: self.list_display.remove('subjectMatter') if 'apply_prove' not in self.list_display: self.list_display.append('apply_prove') qs = qs.values('month', 'warehouse').annotate(apply_prove=Count('id')).distinct() for item in qs: warehouse = warehouseInformation.objects.get(id=item['warehouse']) obj = warehousePeople(month=item['month'], warehouse=warehouse) obj.apply_prove = item['apply_prove'] result.append(obj) 怎么将result转换为QuerySet,并且保留result列表中自定义字段apply_prove 代码应该怎么修改
要将`result`转换为`QuerySet`并保留自定义字段`apply_prove`,可以使用`QuerySet`的`values()`和`annotate()`方法来实现。下面是修改后的代码:
```python
from django.db.models import Count
def get_queryset(self, request):
qs = super().get_queryset(request)
result = []
if 'name' in request.GET and request.GET['name'] != 'none':
# 条件查询
qs = warehousePeople.objects.filter(name=request.GET['name'])
if 'subjectMatter' in self.list_display:
self.list_display.remove('subjectMatter')
if 'apply_prove' not in self.list_display:
self.list_display.append('apply_prove')
qs = qs.values('month', 'warehouse').annotate(apply_prove=Count('id')).distinct()
for item in qs:
warehouse = warehouseInformation.objects.get(id=item['warehouse'])
obj = warehousePeople(month=item['month'], warehouse=warehouse)
obj.apply_prove = item['apply_prove']
result.append(obj)
# 将result转换为QuerySet并保留自定义字段apply_prove
qs = warehousePeople.objects.none()
for obj in result:
qs |= warehousePeople.objects.filter(month=obj.month, warehouse=obj.warehouse).values(*self.list_display).annotate(apply_prove=obj.apply_prove)
return qs
```
这里使用了`qs.values('month', 'warehouse').annotate(apply_prove=Count('id')).distinct()`来计算每个月、每个仓库的`apply_prove`值,并将结果存储在`item['apply_prove']`中。然后,将每个`item`转换为`warehousePeople`对象,并将`apply_prove`值存储在`obj.apply_prove`中。最后,使用`|=`操作符将每个`obj`对应的`QuerySet`合并到`qs`中,并返回`qs`。
相关推荐
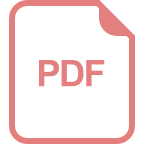
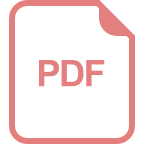
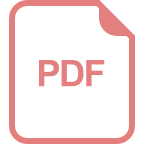












