遍历类属性 python
时间: 2023-10-22 20:18:43 浏览: 253
在Python中,可以通过内置函数`dir()`来获取一个类的所有属性和方法,包括继承的属性和方法。而如果只想获取类的属性,可以使用`vars()`函数,它返回一个字典,其中包含类的所有属性和它们的值。
以下是一个示例代码,演示如何遍历类的属性:
```python
class MyClass:
def __init__(self, x, y):
self.x = x
self.y = y
def my_method(self):
pass
obj = MyClass(1, 2)
print("类属性:")
for attr in vars(MyClass):
print(attr)
print("\n实例属性:")
for attr in vars(obj):
print(attr)
```
输出结果为:
```
类属性:
__module__
__init__
my_method
__dict__
__weakref__
__doc__
实例属性:
x
y
```
可以看到,`vars(MyClass)`返回了包含类属性的字典,而`vars(obj)`返回了包含实例属性的字典。如果想要遍历实例的属性及其值,可以直接对`obj.__dict__`进行遍历。
相关问题
opencv遍历图像像素python
### 回答1:
使用OpenCV库中的函数,可以很容易地遍历图像像素。以下是一个Python示例代码:
```python
import cv2
# 读取图像
img = cv2.imread('image.jpg')
# 获取图像的宽度和高度
height, width = img.shape[:2]
# 遍历图像像素
for y in range(height):
for x in range(width):
# 获取像素值
pixel = img[y, x]
# 处理像素值
# ...
# 显示图像
cv2.imshow('image', img)
cv2.waitKey()
cv2.destroyAllWindows()
```
在上面的代码中,首先使用`cv2.imread()`函数读取图像。然后,使用`img.shape`属性获取图像的宽度和高度。接下来,使用两个嵌套的`for`循环遍历图像的每个像素。在循环中,使用`img[y, x]`获取当前像素的值。最后,可以在循环外部使用`cv2.imshow()`函数显示图像。
### 回答2:
OpenCV是一个开源的计算机视觉库,提供了很多图像处理和计算机视觉功能。在OpenCV中,遍历图像像素是一个非常基础的操作,这个操作在图像处理的许多应用中都非常有用。Python是一种非常流行的编程语言,也是OpenCV支持的一种编程语言。在Python中,OpenCV提供了很多遍历图像像素的函数,这让我们可以很容易地遍历图像像素,进行各种图像处理操作。
在OpenCV中,遍历图像像素的最基本方式是使用for循环,遍历图像的每一个像素,并进行各种操作。以下是一个简单的例子,演示了如何使用for循环在OpenCV中遍历图像像素:
```
import cv2
import numpy as np
# 加载图像
img = cv2.imread('test.jpg')
# 获取图像的宽度和高度
height, width, channels = img.shape
# 遍历图像像素
for row in range(height):
for col in range(width):
# 获取当前像素值
b, g, r = img[row, col]
# 对当前像素进行操作
img[row, col] = [r, g, b]
# 显示处理后的图像
cv2.imshow('result', img)
cv2.waitKey(0)
```
在这个例子中,我们首先加载了一张图像,并使用img.shape获取了图像的宽度、高度和通道数。然后,我们使用两个for循环遍历了整个图像,对每个像素进行了逆序操作,最后使用cv2.imshow显示了处理后的图像。这里需要注意的是,使用for循环遍历图像像素的速度比较慢,如果处理的图像比较大,可能会耗时很长。
除了使用for循环遍历图像像素外,OpenCV还提供了另外一种更快的方式,使用numpy中的数组操作。以下是一个示例代码,演示了如何使用数组操作在OpenCV中遍历图像像素:
```
import cv2
import numpy as np
# 加载图像
img = cv2.imread('test.jpg')
# 使用数组操作逆序图像像素
img = img[:,:,::-1]
# 显示处理后的图像
cv2.imshow('result', img)
cv2.waitKey(0)
```
在这个例子中,我们首先加载了一张图像,并使用数组操作img[:,:,::-1]逆序了图像像素。这里需要注意的是,数组操作的速度要比for循环快得多。因此,如果需要对大型图像进行像素级操作,使用数组操作会更加高效。
总之,在OpenCV中遍历图像像素有很多种方式,使用for循环和数组操作是其中比较常见的两种方式。视情况而定,选择适合自己的方式进行图像处理即可。
### 回答3:
OpenCV是一个开源计算机视觉库,允许开发人员使用Python、C++等语言来进行视觉任务的开发。在图像处理中,遍历图像像素是一个非常基础的操作,也是在OpenCV中经常需要用到的。下面将从以下三个方面,讲解在Python中如何遍历图像像素:
一、遍历方式
在Python中,我们可以使用for循环来遍历图像像素。如果图像格式为单通道的灰度图像,那么每个像素点都是一个0到255范围内的整数值。如果图像格式为RGB图像,那么每个像素点都是由3个颜色分量R、G、B组成的,每个分量也是0到255范围内的整数值。所以,在遍历图像像素之前,我们需要确定当前处理的像素格式是单通道或RGB格式。
二、遍历像素
1.单通道灰度图像遍历
#导入OpenCV模块
import cv2
#读入灰度图像
img_gray=cv2.imread('lena_gray.jpg',cv2.IMREAD_GRAYSCALE)
#获取图像宽高
height,width=img_gray.shape[:2]
#遍历灰度图像像素
for i in range(height):
for j in range(width):
pixel=img_gray[i,j]
print('像素坐标(',i,',',j,') 灰度值:',pixel)
2.RGB图像遍历
#读取RGB图像
img_bgr=cv2.imread('lena_bgr.jpg')
#获取图像宽高
height,width,channels=img_bgr.shape
#遍历RGB图像像素
for i in range(0, height):
for j in range(0, width):
b,g,r=img_bgr[i,j]
print("像素坐标(", i, ",", j, ") [B,G,R]: [", b, ",", g, ",", r, "]")
三、切片遍历
在OpenCV中,利用切片方式也可以方便地遍历图像像素。切片是从一个大数组中取出一个子集,因此可以轻松地访问图像的某个特定区域。对于RGB图像,每个像素包含3个颜色通道,可以单独对每个通道进行切片遍历。
#读取RGB图像
img_bgr=cv2.imread('lena_bgr.jpg')
#选取图像右上角100*100区域
region=img_bgr[0:100,0:100]
#遍历RGB图像像素
for i in range(0, 100):
for j in range(0, 100):
b,g,r=region[i,j]
print("像素坐标(", i, ",", j, ") [B,G,R]: [", b, ",", g, ",", r, "]")
总之,在OpenCV中遍历图像像素是一项基本技能,而Python的简洁语法和强大支持,使得这项任务更加轻松愉快。
Python 遍历xml节点属性
你可以使用Python中的`xml.etree.ElementTree`模块来遍历xml节点属性。以下是一个简单的示例代码:
```python
import xml.etree.ElementTree as ET
# 解析xml文件
tree = ET.parse('example.xml')
# 获取根节点
root = tree.getroot()
# 遍历节点
for child in root:
# 获取节点属性
attribute_value = child.attrib.get('attribute_name')
print(attribute_value)
```
在上面的代码中,我们首先使用`ET.parse`函数解析了一个名为`example.xml`的xml文件。然后,我们使用`getroot()`方法获取xml文件的根节点。接下来,我们使用一个for循环遍历根节点下的所有子节点,并使用`attrib.get()`方法获取每个节点的属性值。
阅读全文
相关推荐
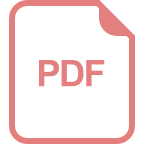
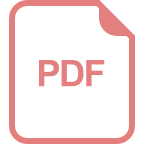












