具体代码Python
时间: 2023-07-10 19:13:57 浏览: 87
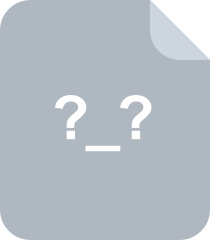
python代码

好的,下面是Python实现LDA主题模型的具体代码:
1. 数据预处理
```python
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
from nltk.stem import WordNetLemmatizer
from gensim.corpora import Dictionary
# 加载停用词
nltk.download('stopwords')
stop_words = set(stopwords.words('english'))
# 加载WordNetLemmatizer词形还原器
nltk.download('wordnet')
lemmatizer = WordNetLemmatizer()
# 文本预处理
def preprocess(text):
# 将文本转化为小写
text = text.lower()
# 分词
tokens = word_tokenize(text)
# 去除停用词和低频词
tokens = [token for token in tokens if token not in stop_words and len(token) > 3]
# 词形还原
tokens = [lemmatizer.lemmatize(token) for token in tokens]
return tokens
# 构建词典
def build_dict(data):
dictionary = Dictionary(data)
dictionary.filter_extremes(no_below=5, no_above=0.5)
return dictionary
```
2. 构建词袋
```python
from gensim.models import TfidfModel
from gensim.matutils import corpus2dense
# 构建词袋模型
def build_corpus(data, dictionary):
corpus = [dictionary.doc2bow(doc) for doc in data]
# 构建tf-idf矩阵
tfidf = TfidfModel(corpus)
corpus_tfidf = tfidf[corpus]
# 将稀疏矩阵转化为稠密矩阵
corpus_dense = corpus2dense(corpus_tfidf, num_terms=len(dictionary)).T
return corpus_dense
```
3. 训练LDA模型
```python
from gensim.models import LdaModel
# 训练LDA模型
def train_lda(corpus, dictionary, num_topics=10, passes=10):
lda = LdaModel(corpus=corpus, id2word=dictionary, num_topics=num_topics, passes=passes)
return lda
```
4. 分析主题
```python
# 查看每个主题中包含的单词
topic_words = lda.show_topics(num_topics=num_topics, num_words=10)
for i in range(num_topics):
print('主题%d:' % i)
print(topic_words[i])
# 查看每个文档所属的主题
doc_topics = lda.get_document_topics(corpus)
for i in range(len(doc_topics)):
print('文档%d的主题分布:' % i)
print(doc_topics[i])
```
5. 应用主题模型
```python
# 对新文本进行主题分类
def predict_topic(lda, dictionary, text):
tokens = preprocess(text)
bow = dictionary.doc2bow(tokens)
topic = lda.get_document_topics(bow)
return topic
```
以上是Python实现LDA主题模型的具体代码,希望能对您有所帮助。
阅读全文
相关推荐
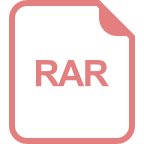
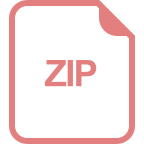
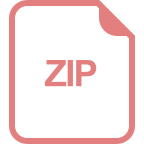
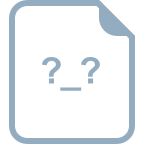
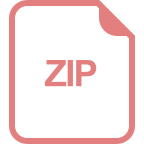
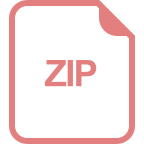
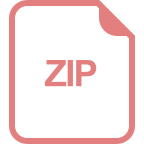
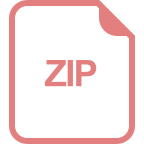
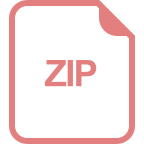
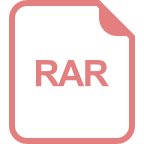
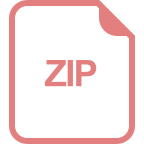
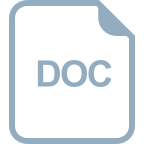
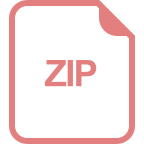
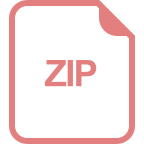
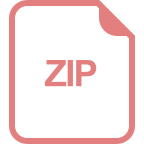
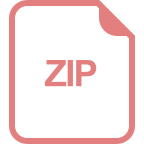