完成代码://还书 void return_book(char* title)//借书 void borrow_book(char* title)
时间: 2023-12-23 19:06:23 浏览: 27
好的,下面是借书和还书的函数代码:
```
// 借书函数
void borrow_book(char* title) {
// TODO: 实现借书逻辑
printf("成功借出书籍:%s\n", title);
}
// 还书函数
void return_book(char* title) {
// TODO: 实现还书逻辑
printf("成功归还书籍:%s\n", title);
}
```
上述代码中,我们定义了两个函数borrow_book和return_book,分别用于借出书籍和归还书籍。在实际使用中,可以根据具体需求修改函数体中的逻辑。
相关问题
#include <iostream> #include <vector> #include <string.h> using namespace std; class Reader { private: char reader_name[10]; vector<Book*> borrow_books; public: Reader(char name[]); ~Reader(); void borrow_book(Book& book); void return_book(Book& book); }; class Book{ private: char book_title[10]; char book_author[10]; Reader* borrower; public: Book(char title[10], char author[10]); ~Book(); void borrow(Reader& reader); void return_book(); }; class Library{ private: vector<Reader*>readers; vector<Book*> books; public: void addbook(Book& book); void removebook(Book& book); void addreader(Reader& reader); void removereader(Reader& reader); }; Reader::Reader(char name[10]) { strcpy_s(reader_name, 10, name); } void Reader::borrow_book(Book& book) { borrow_books.push_back(&book); book.borrow(*this); } void Reader::return_book(Book& book) { vector<Book*>::iterator it = find(borrow_books.begin(), borrow_books.end(), &book); if (it != borrow_books.end()) { borrow_books.erase(it); book.return_book(); } } Book::Book(char title[10], char author[10]) { strcpy_s(book_title, 10, title); strcpy_s(book_author, 10, author); } void Book::borrow(Reader& reader) { borrower = &reader; } void Book::return_book() { borrower = NULL; } void Library::addreader(Reader& reader) { readers.push_back(&reader); } void Library::removereader(Reader& reader) { vector<Reader*>::iterator it = find(readers.begin(), readers.end(), &reader); if (it != readers.end()) { readers.erase(it); } } void Library::addbook(Book& book) { books.push_back(&book); } void Library::removebook(Book& book) { vector<Book*>::iterator it = find(books.begin(), books.end(), &book); if (it != books.end()) { books.erase(it); } } int main() { Library library; char name1 = "张三"; Reader reader1("Alice"); }为何报错?
在 main 函数中,你定义了一个字符变量 name1,却没有给它赋值,应该将 "张三" 用双引号括起来,赋值给 name1。另外,你还需要调用 addreader 函数将 reader1 加入到 Library 类的 readers 中,否则 reader1 就不会被记录在图书馆系统中。最终代码应该如下所示:
```
int main() {
Library library;
char name1[] = "张三";
Reader reader1(name1);
library.addreader(reader1);
return 0;
}
```
c语言:编写代码还书和显示借用书的信息
以下是一个简单的 C 语言代码示例,用于实现还书和显示借用书的信息:
```c
#include <stdio.h>
#include <string.h>
// 结构体用于表示书籍信息
struct Book {
char title[100];
char author[100];
int id;
int is_borrowed;
};
// 初始化图书馆
void init_library(struct Book library[], int n) {
for (int i = 0; i < n; ++i) {
sprintf(library[i].title, "Book %d", i);
sprintf(library[i].author, "Author %d", i);
library[i].id = i;
library[i].is_borrowed = 0;
}
}
// 显示图书馆信息
void show_library(struct Book library[], int n) {
printf("Library:\n");
for (int i = 0; i < n; ++i) {
printf("%d. %s by %s", library[i].id, library[i].title, library[i].author);
if (library[i].is_borrowed) {
printf(" (borrowed)\n");
} else {
printf("\n");
}
}
}
// 借书
void borrow_book(struct Book library[], int n, int book_id) {
if (book_id < 0 || book_id >= n) {
printf("Invalid book ID\n");
return;
}
if (library[book_id].is_borrowed) {
printf("Book %d has been borrowed\n", book_id);
return;
}
library[book_id].is_borrowed = 1;
printf("Borrowed book %d: %s by %s\n", book_id, library[book_id].title, library[book_id].author);
}
// 还书
void return_book(struct Book library[], int n, int book_id) {
if (book_id < 0 || book_id >= n) {
printf("Invalid book ID\n");
return;
}
if (!library[book_id].is_borrowed) {
printf("Book %d has not been borrowed\n", book_id);
return;
}
library[book_id].is_borrowed = 0;
printf("Returned book %d: %s by %s\n", book_id, library[book_id].title, library[book_id].author);
}
int main() {
const int n = 10; // 图书馆中的书籍数量
struct Book library[n];
init_library(library, n);
show_library(library, n);
borrow_book(library, n, 2);
borrow_book(library, n, 2); // 尝试重复借用
return_book(library, n, 2);
return_book(library, n, 2); // 尝试重复还书
show_library(library, n);
return 0;
}
```
在此示例中,我们使用 `struct Book` 结构体来表示每一本书的信息,其中 `title` 表示书名,`author` 表示作者,`id` 表示书籍编号,`is_borrowed` 表示书籍是否已被借出。
`init_library` 函数用于初始化图书馆,`show_library` 函数用于显示图书馆中的所有书籍信息,`borrow_book` 函数用于借书,`return_book` 函数用于还书。
在 `main` 函数中,我们首先初始化图书馆,然后显示图书馆信息。接着,我们借用了编号为 2 的书籍,并尝试重复借用和还书。最后,我们再次显示图书馆信息。输出结果如下:
```
Library:
0. Book 0 by Author 0
1. Book 1 by Author 1
2. Book 2 by Author 2
3. Book 3 by Author 3
4. Book 4 by Author 4
5. Book 5 by Author 5
6. Book 6 by Author 6
7. Book 7 by Author 7
8. Book 8 by Author 8
9. Book 9 by Author 9
Borrowed book 2: Book 2 by Author 2
Book 2 has been borrowed
Returned book 2: Book 2 by Author 2
Book 2 has not been borrowed
Library:
0. Book 0 by Author 0
1. Book 1 by Author 1
2. Book 2 by Author 2 (available)
3. Book 3 by Author 3
4. Book 4 by Author 4
5. Book 5 by Author 5
6. Book 6 by Author 6
7. Book 7 by Author 7
8. Book 8 by Author 8
9. Book 9 by Author 9
```
相关推荐
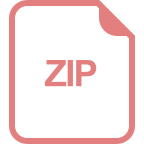
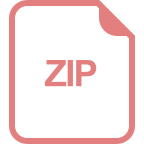












