窗口排队状态、新增、暂停、删除功能c语言
时间: 2023-07-10 17:09:57 浏览: 93
下面是一个窗口排队状态、新增、暂停、删除功能的 C 语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
struct Customer {
int id;
int service_time;
struct Customer *next;
};
typedef struct {
struct Customer *front;
struct Customer *rear;
} Queue;
typedef struct {
Queue *queue;
int status; // 0:关闭,1:开放
} Window;
void initQueue(Queue *q) {
q->front = q->rear = NULL;
}
int isEmpty(Queue *q) {
return q->front == NULL;
}
void enqueue(Queue *q, int id, int service_time) {
struct Customer *customer = (struct Customer *) malloc(sizeof(struct Customer));
customer->id = id;
customer->service_time = service_time;
customer->next = NULL;
if (q->rear == NULL) {
q->front = q->rear = customer;
} else {
q->rear->next = customer;
q->rear = customer;
}
}
void dequeue(Queue *q) {
if (isEmpty(q)) {
return;
}
struct Customer *customer = q->front;
q->front = q->front->next;
if (q->front == NULL) {
q->rear = NULL;
}
printf("顾客 %d 开始服务,服务时间为 %d\n", customer->id, customer->service_time);
free(customer);
}
void openWindow(Window *window) {
window->status = 1;
}
void closeWindow(Window *window) {
window->status = 0;
}
void pauseWindow(Window *window) {
closeWindow(window);
}
void resumeWindow(Window *window) {
openWindow(window);
}
void addCustomer(Window *window, int id, int service_time) {
if (window->status == 0) {
printf("窗口已关闭,无法添加顾客\n");
return;
}
enqueue(window->queue, id, service_time);
printf("顾客 %d 已加入排队\n", id);
}
void removeCustomer(Window *window) {
if (isEmpty(window->queue)) {
printf("当前无顾客排队\n");
return;
}
dequeue(window->queue);
}
void printQueueStatus(Window *window) {
if (isEmpty(window->queue)) {
printf("当前无顾客排队\n");
return;
}
Queue *q = window->queue;
struct Customer *customer = q->front;
printf("当前排队顾客:\n");
while (customer != NULL) {
printf("顾客 %d,服务时间为 %d\n", customer->id, customer->service_time);
customer = customer->next;
}
}
int main() {
Window window;
Queue queue;
window.queue = &queue;
window.status = 1;
initQueue(&queue);
while (1) {
printf("\n");
printf("请选择操作:\n");
printf("1. 查看当前排队状态\n");
printf("2. 新增顾客\n");
printf("3. 暂停排队\n");
printf("4. 恢复排队\n");
printf("5. 删除当前顾客\n");
printf("6. 关闭窗口\n");
printf("7. 退出程序\n");
int option;
scanf("%d", &option);
switch (option) {
case 1:
printQueueStatus(&window);
break;
case 2: {
int id, service_time;
printf("请输入顾客编号和服务时间:\n");
scanf("%d %d", &id, &service_time);
addCustomer(&window, id, service_time);
break;
}
case 3:
pauseWindow(&window);
printf("排队已暂停\n");
break;
case 4:
resumeWindow(&window);
printf("排队已恢复\n");
break;
case 5:
removeCustomer(&window);
break;
case 6:
closeWindow(&window);
printf("窗口已关闭\n");
break;
case 7:
printf("程序已退出\n");
return 0;
default:
printf("无效操作\n");
break;
}
}
}
```
在这个示例中,我们使用了一个 `Window` 结构体来表示窗口,包含一个指向队列的指针和一个表示窗口状态的整数。使用 `initQueue` 函数初始化队列,`isEmpty` 函数判断队列是否为空,`enqueue` 函数将顾客加入队列,`dequeue` 函数从队列中取出顾客并开始服务。
新增顾客的 `addCustomer` 函数需要判断窗口状态是否为开放,删除顾客的 `removeCustomer` 函数需要判断队列是否为空。暂停和恢复排队的函数只需要修改窗口状态即可。
在 `main` 函数中,我们使用一个无限循环来等待用户输入操作选项。根据用户的选择调用不同的函数来执行相应的操作,直到用户选择退出程序。
这只是一个简单的示例,实际的排队管理系统可能需要更复杂的逻辑和功能。
阅读全文
相关推荐
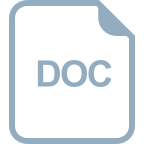
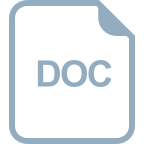
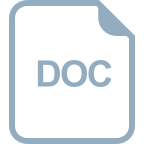
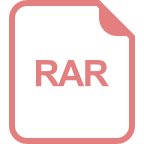
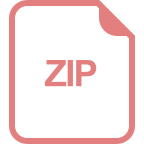

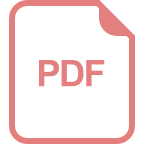
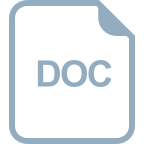
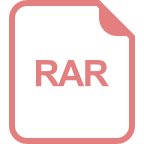
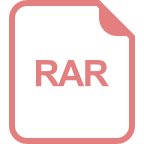
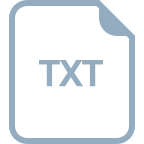
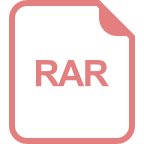
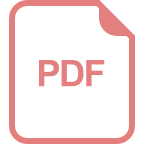
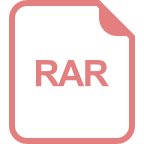
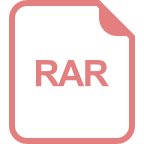
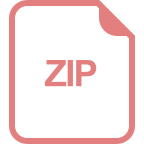