演示一下strstr函数
时间: 2023-10-12 22:11:20 浏览: 50
strstr函数是C语言中的字符串函数,用于在一个字符串中查找另一个子字符串的位置。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "hello, world!";
char *ptr = strstr(str, "world");
if (ptr != NULL) {
printf("Substring found at position %d.\n", ptr - str);
} else {
printf("Substring not found.\n");
}
return 0;
}
```
以上代码会在字符串“hello, world!”中查找子字符串“world”,并输出其位置。如果找到了,则会输出“Substring found at position x.”,其中x是子字符串在原字符串中的起始位置。如果没有找到,则会输出“Substring not found.”。
输出结果为:
```
Substring found at position 7.
```
相关问题
strstr函数讲解
strstr函数是C/C++语言中的一个字符串处理函数,其作用是在一个字符串中查找另一个指定的子字符串,并返回该子字符串在原字符串中的位置。
函数原型如下:
```c++
char *strstr(const char *str1, const char *str2);
```
其中,str1表示要在其中查找子字符串的字符串,str2表示要查找的子字符串。
函数返回值:
如果查找成功,则返回str1中第一次出现str2的位置(即str2在str1中第一次出现的位置)的指针。
如果查找失败,则返回空指针。
例如,下面的代码演示了使用strstr函数查找子字符串的示例:
```c++
#include <stdio.h>
#include <string.h>
int main()
{
char str1[] = "Hello, world!";
char str2[] = "world";
char *p = strstr(str1, str2);
if (p != NULL)
{
printf("Found: %s\n", p);
}
else
{
printf("Not found.\n");
}
return 0;
}
```
输出结果为:
```
Found: world!
```
这个例子中,我们在字符串str1中查找字符串str2,由于str2出现在str1中,因此函数返回了指向“world”这个子字符串的指针。
c语言strstr函数原型
C语言中的strstr函数原型如下:
```c
char *strstr(const char *haystack, const char *needle);
```
该函数用于在字符串haystack中查找子串needle,并返回第一次出现的位置的指针。如果未找到该子串,则返回空指针。
其中,haystack表示要查找的字符串,needle表示要查找的子串。
以下是一个示例代码,演示了如何使用strstr函数查找子串:
```c
#include <stdio.h>
#include <string.h>
int main () {
const char haystack[20] = "Hello World";
const char needle[10] = "World";
char *ret;
ret = strstr(haystack, needle);
printf("The substring is: %s\n", ret);
return(0);
}
```
输出结果为:
```
The substring is: World
```
阅读全文
相关推荐
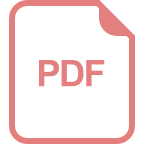









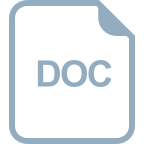


