nodejs实现将zip文件转化为流的形式传给主进程进行下载
时间: 2023-11-28 09:47:14 浏览: 87
可以使用 `fs.createReadStream` 和 `archiver` 模块来实现将 zip 文件转化为流的形式传给主进程进行下载。
以下是实现的代码示例:
```javascript
const fs = require('fs');
const archiver = require('archiver');
const zipFilePath = 'path/to/your/zip/file';
// 创建一个可写流
const stream = fs.createWriteStream('path/to/your/output/file.zip');
// 创建一个压缩器
const archive = archiver('zip', {
zlib: { level: 9 } // 设置压缩级别
});
// 监听压缩器的 'warning' 和 'error' 事件
archive.on('warning', function(err) {
if (err.code === 'ENOENT') {
console.warn('archiver warning: ', err);
} else {
throw err;
}
});
archive.on('error', function(err) {
throw err;
});
// 将 zip 文件添加到压缩器中
archive.file(zipFilePath, { name: 'your-zip-file.zip' });
// 将压缩器的输出流 pipe 到可写流中
archive.pipe(stream);
// 完成压缩并关闭压缩器和可写流
archive.finalize();
stream.on('close', function() {
console.log('Zip file has been streamed and downloaded successfully.');
});
```
在主进程中,你可以使用 Node.js 的 `fs` 模块和 `http` 或 `https` 模块来将流写入响应中,以供用户下载。以下是一个 HTTP 服务器的示例:
```javascript
const http = require('http');
const fs = require('fs');
http.createServer(function(req, res) {
const filePath = 'path/to/your/output/file.zip';
const stat = fs.statSync(filePath);
res.writeHead(200, {
'Content-Type': 'application/zip',
'Content-Length': stat.size,
'Content-Disposition': 'attachment; filename=your-zip-file.zip'
});
const stream = fs.createReadStream(filePath);
stream.pipe(res);
stream.on('error', function(err) {
console.error(err);
res.statusCode = 500;
res.end('Error while streaming the file.');
});
}).listen(3000, function() {
console.log('Server listening on port 3000.');
});
```
当用户访问 `http://your-server-url:3000` 时,浏览器会自动下载 `your-zip-file.zip` 文件。
阅读全文
相关推荐
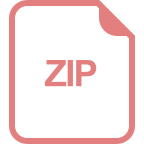
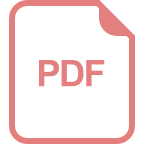
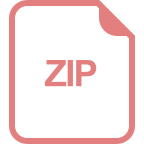
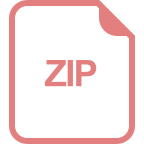
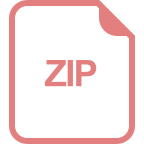
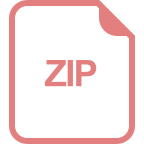
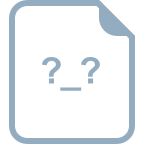
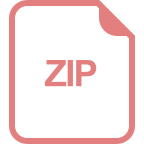
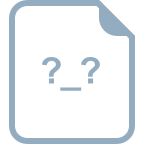
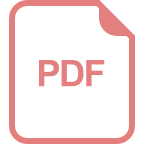
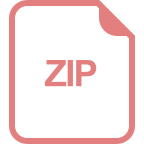
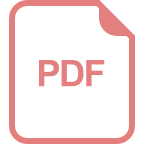
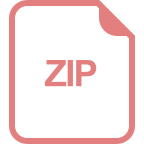