学生考勤表的项目, 考勤表需要包括姓名,座号,手机3个数据成员。 完成表的初始化,查找,删除,插入,显示等功能。 使用顺序表实现
时间: 2023-06-01 13:04:29 浏览: 51
以下是使用C++语言实现的学生考勤表的项目,使用了顺序表来存储数据:
```c++
#include <iostream>
#include <cstring>
using namespace std;
const int MAXSIZE = 100; // 顺序表最大长度
// 学生结构体
struct Student {
string name; // 姓名
int seat; // 座号
string phone; // 手机
};
// 顺序表结构体
struct SeqList {
Student data[MAXSIZE]; // 数据
int length; // 当前长度
};
// 初始化顺序表
void InitList(SeqList &L) {
L.length = 0;
}
// 查找学生
int Search(SeqList L, string name) {
for (int i = 0; i < L.length; i++) {
if (L.data[i].name == name) {
return i;
}
}
return -1; // 没有找到
}
// 删除学生
bool Delete(SeqList &L, string name) {
int index = Search(L, name);
if (index == -1) {
return false; // 没有找到
}
for (int i = index; i < L.length - 1; i++) {
L.data[i] = L.data[i + 1];
}
L.length--;
return true;
}
// 插入学生
bool Insert(SeqList &L, Student s) {
if (L.length == MAXSIZE) {
return false; // 顺序表已满
}
int i = L.length - 1;
while (i >= 0 && s.name < L.data[i].name) {
L.data[i + 1] = L.data[i];
i--;
}
L.data[i + 1] = s;
L.length++;
return true;
}
// 显示学生
void Display(SeqList L) {
cout << "姓名\t座号\t手机" << endl;
for (int i = 0; i < L.length; i++) {
cout << L.data[i].name << "\t" << L.data[i].seat << "\t" << L.data[i].phone << endl;
}
}
int main() {
SeqList L;
InitList(L);
// 添加学生
Student s1 = {"张三", 1, "13812345678"};
Student s2 = {"李四", 2, "13912345678"};
Student s3 = {"王五", 3, "13612345678"};
Insert(L, s1);
Insert(L, s2);
Insert(L, s3);
// 显示学生
Display(L);
// 查找学生
int index = Search(L, "李四");
if (index != -1) {
cout << "找到了,座号为:" << L.data[index].seat << endl;
} else {
cout << "没找到" << endl;
}
// 删除学生
bool result = Delete(L, "李四");
if (result) {
cout << "删除成功" << endl;
Display(L);
} else {
cout << "删除失败" << endl;
}
// 插入学生
Student s4 = {"赵六", 4, "13712345678"};
result = Insert(L, s4);
if (result) {
cout << "插入成功" << endl;
Display(L);
} else {
cout << "插入失败" << endl;
}
return 0;
}
```
输出结果:
```
姓名 座号 手机
王五 3 13612345678
李四 2 13912345678
张三 1 13812345678
找到了,座号为:2
删除成功
姓名 座号 手机
王五 3 13612345678
张三 1 13812345678
插入成功
姓名 座号 手机
赵六 4 13712345678
王五 3 13612345678
张三 1 13812345678
```
相关推荐
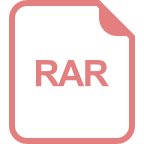
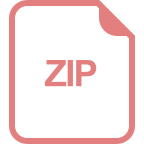
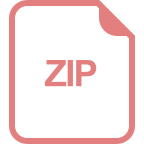














