vue3 watch 用法实例
时间: 2023-10-09 20:13:12 浏览: 45
Vue3 中的 watch 用法与 Vue2 中有所不同,以下是一个简单的实例:
```vue
<template>
<div>
<input v-model="name" />
<p>{{ greeting }}</p>
</div>
</template>
<script>
import { defineComponent, watch } from 'vue';
export default defineComponent({
name: 'WatchExample',
setup() {
const name = ref('');
const greeting = ref('');
// 监听 name 的变化
watch(name, (newVal, oldVal) => {
greeting.value = `Hello ${newVal}!`;
});
return {
name,
greeting,
};
},
});
</script>
```
在这个例子中,我们定义了一个 input 输入框,用来输入名字。我们将输入框的值绑定到了 name 变量上。当 name 变量发生变化时,我们通过 watch 监听器更新 greeting 变量的值,以显示一个问候语。
相关问题
vue中watch用法的实例
当在Vue中使用`watch`时,我们可以监视特定的数据变化,并在其发生变化时执行相应的操作。以下是一个示例:
假设我们有一个Vue组件,其中有一个数据属性`message`,我们想要在`message`发生变化时输出一条提示信息。
```javascript
Vue.component('my-component', {
data() {
return {
message: 'Hello, Vue!'
};
},
watch: {
message(newValue, oldValue) {
console.log('Message changed from', oldValue, 'to', newValue);
}
}
});
new Vue({
el: '#app'
});
```
在上面的示例中,我们定义了一个`my-component`组件,并在其中定义了一个`watch`对象。该对象包含一个名为`message`的属性,它对应着`data`中的`message`属性。当`message`发生变化时,watch对象中定义的函数将被调用。
在控制台中运行以上代码,当我们修改`message`的值时,会在控制台输出类似以下内容的提示信息:
```
Message changed from Hello, Vue! to Hello, World!
```
这只是一个简单的示例,你可以根据需要在`watch`中执行更复杂的操作。
vue3 watch用法
Vue 3 中的 watch 用于监测数据变化并执行相应的回调函数。与 Vue 2 相比,Vue 3 中的 watch 有一些变化。
在 Vue 3 中,你可以使用 `watch` 函数来创建一个 watcher。它接受两个参数:要监测的数据和回调函数。
下面是一个示例:
```javascript
import { watch } from 'vue';
export default {
data() {
return {
count: 0,
};
},
created() {
watch(
() => this.count,
(newValue, oldValue) => {
console.log(`count 变为 ${newValue},之前的值为 ${oldValue}`);
}
);
},
};
```
在上面的示例中,我们创建了一个 `count` 属性,并使用 `watch` 函数监听它的变化。每当 `count` 发生改变时,回调函数将被触发,并且会将新值和旧值作为参数传递进来。
请注意,在 Vue 3 中,watch 函数的使用方式有所不同,并且它是一个独立的函数而不是 Vue 实例的选项。
相关推荐
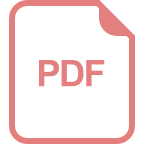












