请用C++语言写一个LCD1602显示字符串的驱动程序
时间: 2024-05-16 19:12:41 浏览: 13
下面是一个简单的LCD1602显示字符串的驱动程序,需要配合相应的硬件电路使用:
```c
#include <reg52.h>
// 定义LCD1602的引脚
sbit RS = P2^0;
sbit RW = P2^1;
sbit EN = P2^2;
#define LCD1602_DATA P0
void delay(unsigned int t) {
while(t--);
}
void write_command(unsigned char command) {
RS = 0; // 设置为写指令模式
RW = 0; // 设置为写模式
LCD1602_DATA = command; // 发送指令
EN = 1; // 使能
delay(10);
EN = 0; // 禁能
}
void write_data(unsigned char data) {
RS = 1; // 设置为写数据模式
RW = 0; // 设置为写模式
LCD1602_DATA = data; // 发送数据
EN = 1; // 使能
delay(10);
EN = 0; // 禁能
}
void init_lcd1602() {
write_command(0x38); // 8位数据总线,双行显示,5x8点阵字符
write_command(0x0c); // 显示开,光标不显示,光标闪烁不显示
write_command(0x06); // 光标右移,字符不移动
write_command(0x01); // 清屏
}
void display_string(unsigned char row, unsigned char col, unsigned char *str) {
unsigned char address;
if(row == 0) {
address = 0x80 + col;
}
else {
address = 0xc0 + col;
}
write_command(address); // 设置显示地址
while(*str) {
write_data(*str++); // 逐个显示字符
}
}
void main() {
init_lcd1602(); // 初始化LCD1602
display_string(0, 0, "Hello, world!"); // 在第一行第一列显示字符串
display_string(1, 0, "Welcome to CSDN!"); // 在第二行第一列显示字符串
while(1); // 死循环
}
```
上述代码中,我们先定义了LCD1602的引脚,并定义了一些函数来实现写指令、写数据、初始化、显示字符串等操作。在主函数中,我们先初始化LCD1602,然后调用`display_string`函数在LCD1602上显示字符串。最后进入死循环。
相关推荐
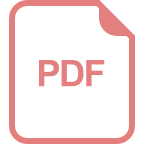
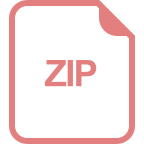
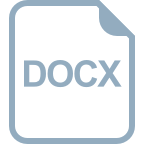









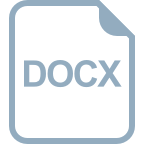
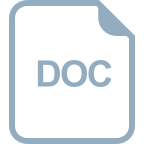
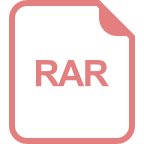
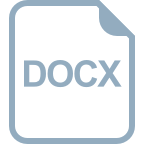
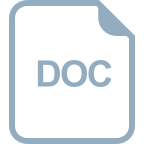
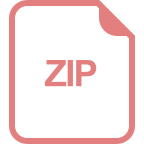