请给出java中在窗体设置字体选择菜单的程序代码,要包括多种字体样式
时间: 2024-01-24 12:16:48 浏览: 73
以下是一个简单的 Java 程序,可以在窗体中设置字体选择菜单,并支持多种字体样式:
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class FontChooser extends JFrame implements ActionListener {
private JComboBox fontBox;
private JComboBox styleBox;
private JComboBox sizeBox;
private JLabel previewLabel;
public FontChooser() {
super("Font Chooser");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Create the font selection controls
String[] fontNames = GraphicsEnvironment.getLocalGraphicsEnvironment().getAvailableFontFamilyNames();
fontBox = new JComboBox(fontNames);
fontBox.addActionListener(this);
String[] styleNames = {"Plain", "Bold", "Italic", "Bold Italic"};
styleBox = new JComboBox(styleNames);
styleBox.addActionListener(this);
String[] sizeNames = {"8", "9", "10", "11", "12", "14", "16", "18", "20", "22", "24", "26", "28", "36", "48", "72"};
sizeBox = new JComboBox(sizeNames);
sizeBox.addActionListener(this);
// Create the preview label
previewLabel = new JLabel("The quick brown fox jumps over the lazy dog.");
previewLabel.setHorizontalAlignment(JLabel.CENTER);
previewLabel.setVerticalAlignment(JLabel.CENTER);
previewLabel.setFont(new Font((String)fontBox.getSelectedItem(), styleBox.getSelectedIndex(), Integer.parseInt((String)sizeBox.getSelectedItem())));
// Create the layout
JPanel controlPanel = new JPanel(new GridLayout(1, 3));
controlPanel.add(fontBox);
controlPanel.add(styleBox);
controlPanel.add(sizeBox);
JPanel previewPanel = new JPanel(new BorderLayout());
previewPanel.add(previewLabel, BorderLayout.CENTER);
setLayout(new BorderLayout());
add(controlPanel, BorderLayout.NORTH);
add(previewPanel, BorderLayout.CENTER);
// Set the initial window size and show the window
setSize(400, 200);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
previewLabel.setFont(new Font((String)fontBox.getSelectedItem(), styleBox.getSelectedIndex(), Integer.parseInt((String)sizeBox.getSelectedItem())));
}
public static void main(String[] args) {
new FontChooser();
}
}
```
这个程序使用了 Java 的 Swing 库,创建了一个窗体,包含了字体选择菜单和一个预览标签。当用户改变任何一个字体选择选项时,程序会更新预览标签的字体。
阅读全文
相关推荐
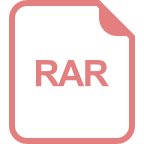

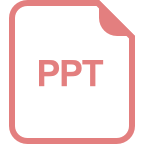
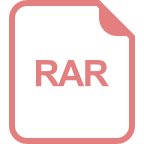
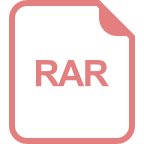
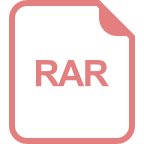
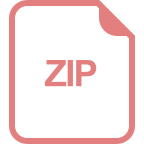
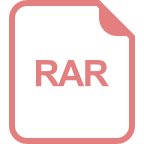
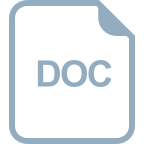
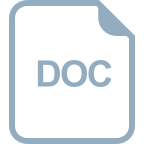
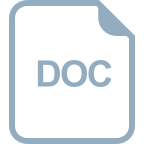
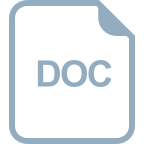
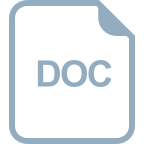
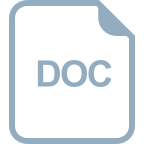
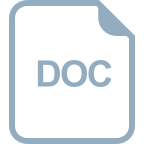
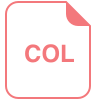