C语言写一个程序输入你看过的一部电影以及每部电影的名称和评分
时间: 2024-05-07 15:22:31 浏览: 137
下面是一个简单的 C 语言程序,用于输入电影名称和评分,并将其存储在一个结构体数组中:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_MOVIES 100
struct Movie {
char title[100];
int rating;
};
int main() {
struct Movie movies[MAX_MOVIES];
int count = 0;
printf("Enter the title and rating of each movie you've seen.\n");
printf("Enter a blank line to stop.\n\n");
while (count < MAX_MOVIES) {
printf("Movie title: ");
fgets(movies[count].title, 100, stdin);
// remove newline character
movies[count].title[strcspn(movies[count].title, "\n")] = 0;
if (movies[count].title[0] == '\0') {
break;
}
printf("Rating (1-10): ");
scanf("%d", &movies[count].rating);
getchar(); // remove newline character
count++;
}
printf("\nYou have entered the following movies:\n\n");
for (int i = 0; i < count; i++) {
printf("%s, %d\n", movies[i].title, movies[i].rating);
}
return 0;
}
```
该程序使用一个结构体 `Movie` 来表示每个电影,包含电影名称和评分。它使用一个结构体数组 `movies` 来存储所有输入的电影。`count` 记录输入的电影数量,最多不超过 `MAX_MOVIES`。
程序使用 `fgets()` 函数来输入电影名称,因为它可以读取包含空格的字符串。然后,程序使用 `strcspn()` 函数来删除字符串末尾的换行符。接着,程序使用 `scanf()` 函数来输入电影评分。最后,程序使用 `getchar()` 函数来删除输入缓冲区中的换行符。
程序输出所有输入的电影名称和评分。
阅读全文
相关推荐
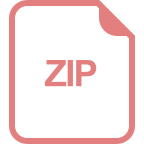
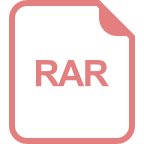
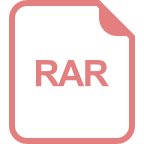
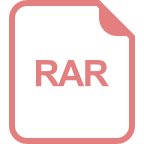
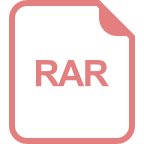
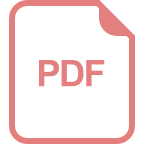
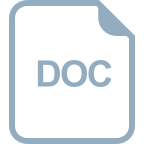
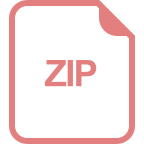
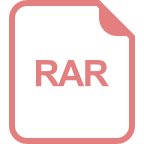
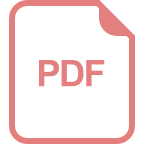