使用Netty写一个server
时间: 2024-02-05 21:12:06 浏览: 89
好的,下面是一个简单的使用Netty写的服务器:
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class NettyServer {
private final int port;
public NettyServer(int port) {
this.port = port;
}
public void run() throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new NettyServerHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
ChannelFuture f = b.bind(port).sync();
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
int port = 8080;
new NettyServer(port).run();
}
}
```
在这个例子中,我们使用了NioEventLoopGroup来处理事件,包括接收连接、读取数据和发送数据。ServerBootstrap是一个用于启动服务器的帮助类。我们使用NioServerSocketChannel作为服务器的通道实现,它允许我们使用NIO来接收新连接。我们还设置了一些选项,如SO_BACKLOG和SO_KEEPALIVE,用于配置服务器。最后,我们将服务器绑定到指定的端口上,并等待直到服务器关闭。在这个例子中,我们使用NettyServerHandler来处理连接,你可以根据需要自己实现。
阅读全文
相关推荐
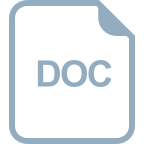
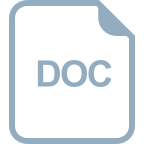
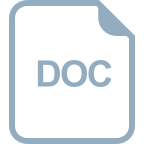
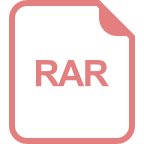
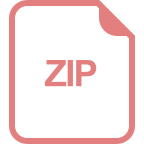
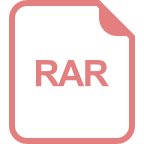
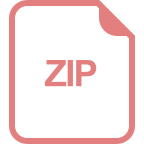
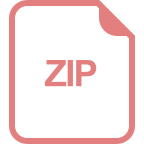
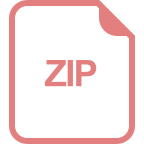
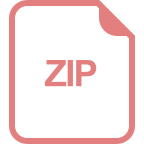








