qt实现socket通信
时间: 2023-06-05 22:04:42 浏览: 68
好的,我可以回答这个问题。QT可以使用QTcpSocket类和QTcpServer类实现socket通信,QTcpSocket用于客户端与服务器之间的通信,QTcpServer用于监听和接受客户端连接。您可以使用QTcpSocket的connectToHost函数连接到服务器,然后使用read和write函数发送和接收数据。详细的实现可以参考QT官方文档或者相关的教程。
相关问题
QT实现socket tcp通信流程
Qt 实现 Socket TCP 通信的流程如下:
1. 创建 QTcpSocket 对象:在需要使用 Socket 的类中,创建 QTcpSocket 对象并设置相关属性,如连接地址、端口号等。
2. 连接服务器:使用 QTcpSocket 的 connectToHost() 函数连接服务器。
3. 发送数据:使用 QTcpSocket 的 write() 函数发送数据。
4. 接收数据:使用 QTcpSocket 的 readyRead() 信号接收数据,然后使用 read() 函数读取数据。
5. 断开连接:使用 QTcpSocket 的 disconnectFromHost() 函数断开与服务器的连接。
下面是一个简单的 QTcpSocket 示例代码:
```cpp
QTcpSocket *socket = new QTcpSocket(this);
socket->connectToHost("192.168.0.1", 8888);
connect(socket, SIGNAL(readyRead()), this, SLOT(readData()));
connect(socket, &QTcpSocket::disconnected, socket, &QTcpSocket::deleteLater);
void MyClass::readData() {
QByteArray data = socket->readAll();
// 处理接收到的数据
}
void MyClass::sendData() {
QByteArray data = "Hello World!";
socket->write(data);
}
void MyClass::disconnect() {
socket->disconnectFromHost();
}
```
这是一个简单的实现,具体的实现还需要根据实际需求来进行调整。
qt实现win socket通信
在Qt中,可以使用QTcpSocket和QTcpServer类来实现Windows Socket通信。下面是一个简单的示例代码:
```cpp
// 创建socket server
QTcpServer *server = new QTcpServer(this);
connect(server, SIGNAL(newConnection()), this, SLOT(onNewConnection()));
if (!server->listen(QHostAddress::Any, 1234)) {
qDebug() << "Error: Failed to start server";
}
// 新连接处理
void MyServer::onNewConnection() {
QTcpSocket *socket = server->nextPendingConnection();
connect(socket, SIGNAL(readyRead()), this, SLOT(onReadyRead()));
connect(socket, SIGNAL(disconnected()), socket, SLOT(deleteLater()));
}
// 读取数据
void MyServer::onReadyRead() {
QTcpSocket *socket = qobject_cast<QTcpSocket *>(sender());
if (!socket) {
return;
}
QByteArray data = socket->readAll();
// 处理数据
// ...
}
```
这里创建了一个QTcpServer对象,并监听所有的IP地址和端口号为1234的连接请求。当有新的连接请求时,会触发newConnection信号,并在onNewConnection函数中创建一个QTcpSocket对象,并连接readyRead信号和disconnected信号。当数据准备好时,会触发readyRead信号,并在onReadyRead函数中读取数据并进行处理。
在客户端,可以使用QTcpSocket类来连接服务器并发送数据。示例代码如下:
```cpp
QTcpSocket *socket = new QTcpSocket(this);
socket->connectToHost("127.0.0.1", 1234);
if (socket->waitForConnected()) {
// 连接成功
socket->write("Hello World");
socket->flush();
}
socket->close();
```
这里创建了一个QTcpSocket对象,并连接到IP地址为127.0.0.1,端口号为1234的服务器。如果连接成功,则发送一个字符串“Hello World”。注意,Qt中的Socket通信是异步的,因此需要等待连接成功后再发送数据。最后需要关闭socket连接。
以上是一个简单的示例,实际使用中需要根据具体需求进行修改。
阅读全文
相关推荐
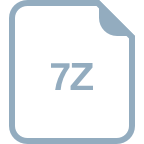
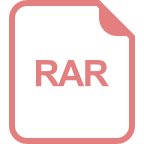
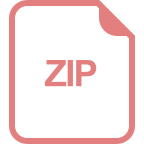
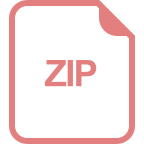
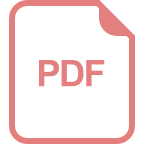


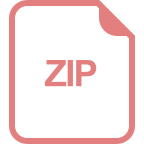
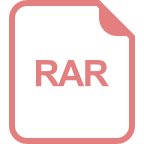



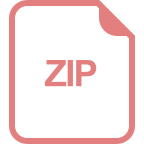